输入一行字符,统计其中有多少个单词(空格分隔),并将the这个单词替换成and后输出。注:此题在做的时候先统计单词个数,然后再替换。
时间: 2023-08-31 22:38:01 浏览: 66
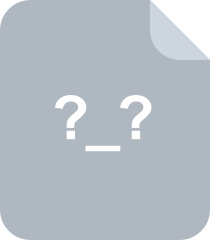
从键盘上输入一串英文字符(不含空格与其他字符),统计每个字符的数目,并输出字母及相应的数目。
### 回答1:
可以使用 Python 来实现此功能,代码如下:
```python
s = input("请输入一行字符:")
words = s.split() # 用空格分隔字符串得到单词列表
count = len(words) # 统计单词个数
s = s.replace("the", "and") # 将 the 替换成 and
print("单词个数:", count)
print("替换后的字符串:", s)
```
例如,输入字符串 "This is the test string.",输出结果为:
```
单词个数: 5
替换后的字符串: This is and test string.
```
### 回答2:
首先,我们可以使用Python的split()函数来将输入的一行字符按照空格分隔成一个单词列表。然后,我们可以使用len()函数统计单词列表中的元素个数,即单词个数。
接下来,我们可以使用replace()函数将单词列表中的"the"替换为"and"。最后,我们可以通过使用join()函数将单词列表中的单词重新连接成一行字符,并输出结果。
以下是一个示例代码:
```python
line = input("请输入一行字符:") # 输入一行字符
words = line.split() # 将字符按照空格分隔成单词列表
word_count = len(words) # 统计单词个数
words = [word.replace("the", "and") for word in words] # 将单词列表中的"the"替换为"and"
output = " ".join(words) # 将单词列表中的单词重新连接成一行字符
print("单词个数:", word_count) # 输出单词个数
print("替换后的字符:", output) # 输出替换后的字符
```
这样,我们就可以通过统计单词个数和替换"the"为"and"来实现题目要求。
### 回答3:
题目要求先统计输入的字符中有多少个单词(以空格分隔),然后将其中的 "the" 替换成 "and" 后输出。
首先,我们可以使用 Python 编程语言来解决这个问题。可以通过以下步骤来实现:
1. 提示用户输入一行字符,并将其存储在一个变量(例如,input_str)中。
2. 初始化一个变量 count,用于记录单词的数量,将其设置为 0。
3. 使用 split() 方法将 input_str 字符串分割成单词,并将结果存储在一个列表(例如,word_list)中。
4. 遍历 word_list 列表中的每个单词:
- 如果当前单词是 "the",则将其替换为 "and",并将 count 增加 1。
- 否则,将 count 增加 1。
5. 打印 count 的值。
6. 使用 join() 方法将修改后的 word_list 列表中的单词重新组合成一个字符串,并使用空格作为分隔符。
7. 打印该字符串。
以下是使用 Python 编写的示例代码:
```python
# 步骤 1
input_str = input("请输入一行字符:")
# 步骤 2
count = 0
# 步骤 3
word_list = input_str.split()
# 步骤 4
for i in range(len(word_list)):
if word_list[i] == "the":
word_list[i] = "and"
count += 1
else:
count += 1
# 步骤 5
print("单词数量:", count)
# 步骤 6
output_str = " ".join(word_list)
# 步骤 7
print("替换后的字符串:", output_str)
```
通过运行上述代码,我们可以实现对输入字符串的单词数量的统计,并将其中的 "the" 替换为 "and" 后输出。
阅读全文
相关推荐
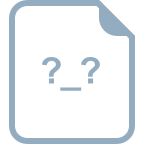















