写一段,图片压缩方法代码,Java语言
时间: 2023-09-04 21:04:05 浏览: 56
### 回答1:
,可以压缩图片的大小。
Here's an example of a Java code that can be used to compress the size of an image:
```
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageCompressor {
public static void main(String[] args) throws IOException {
File input = new File("input.jpg");
BufferedImage image = ImageIO.read(input);
File output = new File("output.jpg");
ImageIO.write(image, "jpg", output);
}
}
```
This code reads an image file and then writes it back out as a new file. The quality of the output image can be adjusted by changing the parameters in the `ImageIO.write` method.
### 回答2:
图片压缩是在保持图片质量的前提下减小图片文件大小的过程。在Java语言中,可以使用ImageIO类和BufferedImage类来实现图片的压缩。
一种常用的图片压缩方法是将图片的分辨率进行缩小,可以通过设置目标图片的宽高来实现。以下是一个简单的图片压缩方法的示例代码:
```java
import java.awt.Image;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageCompressor {
public static void compressImage(String inputImagePath, String outputImagePath, int newWidth, int newHeight) throws IOException {
File inputFile = new File(inputImagePath);
BufferedImage inputImage = ImageIO.read(inputFile);
// 创建一个新的缩放后的图片
Image scaledImage = inputImage.getScaledInstance(newWidth, newHeight, Image.SCALE_DEFAULT);
BufferedImage outputImage = new BufferedImage(newWidth, newHeight, BufferedImage.TYPE_INT_RGB);
// 将缩放后的图像绘制到新的BufferedImage对象上
outputImage.getGraphics().drawImage(scaledImage, 0, 0, null);
// 保存缩放后的图像到输出路径
ImageIO.write(outputImage, "jpg", new File(outputImagePath));
}
}
```
上述代码通过调用`compressImage`方法实现图片的压缩。需要传入参数包括输入图片路径、输出图片路径,以及缩放后的新宽度和高度。该方法使用`ImageIO`类读取输入图片,并使用`BufferedImage`类生成新的缩放后的图片对象。最后,将缩放后的图像保存到输出路径。
通过使用该代码,可以实现对输入图片进行压缩,减小图片文件大小,同时保持图片质量。
### 回答3:
下面是一个使用Java语言编写的简单图片压缩方法的代码:
```java
import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageCompressor {
public static void main(String[] args) {
String inputImagePath = "input.jpg"; // 输入图片路径
String outputImagePath = "output.jpg"; // 压缩后的输出图片路径
int compressedWidth = 800; // 压缩后的图片宽度
int compressedHeight = 600; // 压缩后的图片高度
compressImage(inputImagePath, outputImagePath, compressedWidth, compressedHeight);
}
public static void compressImage(String inputImagePath, String outputImagePath, int compressedWidth, int compressedHeight) {
try {
File inputFile = new File(inputImagePath);
BufferedImage inputImage = ImageIO.read(inputFile);
Image outputImage = inputImage.getScaledInstance(compressedWidth, compressedHeight, Image.SCALE_SMOOTH);
BufferedImage compressedImage = new BufferedImage(compressedWidth, compressedHeight, BufferedImage.TYPE_INT_RGB);
Graphics2D g2d = compressedImage.createGraphics();
g2d.drawImage(outputImage, 0, 0, null);
g2d.dispose();
File outputFile = new File(outputImagePath);
ImageIO.write(compressedImage, "jpg", outputFile);
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
```
这段代码使用`javax.imageio.ImageIO`类库读取输入的图片,然后通过调整图片的宽度和高度来进行压缩。在这个例子中,输入图片的路径为`input.jpg`,压缩后的输出图片路径为`output.jpg`,压缩后的宽度为800像素,高度为600像素。最终的压缩图片将使用`ImageIO.write`方法保存到指定路径。
相关推荐
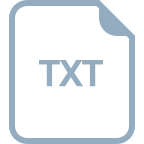
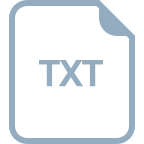
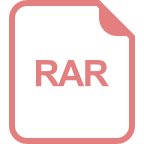














