用java写一段压缩加密文件夹的代码
时间: 2024-02-24 13:00:29 浏览: 19
以下是一个Java代码示例,可以对文件夹进行压缩和加密:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
import javax.crypto.Cipher;
import javax.crypto.CipherOutputStream;
import javax.crypto.spec.SecretKeySpec;
public class FolderCompressor {
private static final String PASSWORD = "myPassword"; // 加密密码
private static final int BUFFER_SIZE = 4096;
public static void compressAndEncryptFolder(String sourceFolder, String outputZipFile) throws Exception {
// 创建输出流
FileOutputStream fos = new FileOutputStream(outputZipFile);
// 创建Zip输出流
ZipOutputStream zos = new ZipOutputStream(fos);
// 设置加密密码
SecretKeySpec key = new SecretKeySpec(PASSWORD.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
// 将加密输出流封装到Zip输出流中
CipherOutputStream cos = new CipherOutputStream(zos, cipher);
// 压缩文件夹
addFolderToZip(sourceFolder, sourceFolder, cos);
// 关闭流
cos.close();
zos.close();
fos.close();
}
private static void addFolderToZip(String rootFolder, String sourceFolder, CipherOutputStream cos) throws Exception {
File folder = new File(sourceFolder);
for (String fileName : folder.list()) {
String filePath = sourceFolder + File.separator + fileName;
if (new File(filePath).isDirectory()) {
// 如果是文件夹,递归调用
addFolderToZip(rootFolder, filePath, cos);
} else {
// 如果是文件,加入Zip输出流
ZipEntry zipEntry = new ZipEntry(filePath.substring(rootFolder.length() + 1));
cos.putNextEntry(zipEntry);
FileInputStream fis = new FileInputStream(filePath);
byte[] buffer = new byte[BUFFER_SIZE];
int length;
while ((length = fis.read(buffer)) > 0) {
cos.write(buffer, 0, length);
}
fis.close();
}
}
}
}
```
使用时,只需要调用 `compressAndEncryptFolder` 方法,并传入要压缩加密的文件夹路径和输出Zip文件路径即可:
```java
FolderCompressor.compressAndEncryptFolder("path/to/source/folder", "path/to/output/file.zip");
```
需要注意的是,这里使用了AES算法进行加密,加密密码为"myPassword"。如果需要更高的安全性,可以考虑使用其他加密算法和更复杂的密码。
相关推荐
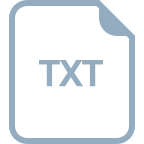
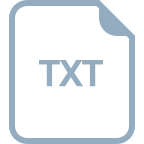
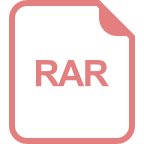














