flutter网络请求
时间: 2023-09-09 12:13:27 浏览: 117
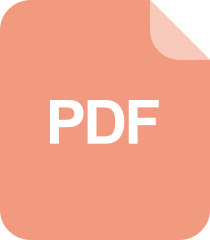
Flutter实现网络请求的方法示例

Flutter 中进行网络请求可以使用 `http` 或者 `dio` 等第三方库。
#### 使用 http
首先,在 `pubspec.yaml` 文件中添加依赖:
```
dependencies:
http: ^0.13.3
```
然后,在需要进行网络请求的地方引入 `http` 库:
```dart
import 'package:http/http.dart' as http;
```
接下来,可以使用 `http.get` 或者 `http.post` 方法进行 GET 或者 POST 请求。例如,使用 GET 方法请求一个 JSON 数据:
```dart
Future<void> fetchAlbum() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/albums/1'));
if (response.statusCode == 200) {
// 请求成功,处理响应数据
print(response.body);
} else {
// 请求失败,处理错误信息
print('请求失败,状态码为 ${response.statusCode}');
}
}
```
#### 使用 dio
首先,在 `pubspec.yaml` 文件中添加依赖:
```
dependencies:
dio: ^4.0.0
```
然后,在需要进行网络请求的地方引入 `dio` 库:
```dart
import 'package:dio/dio.dart';
```
接下来,可以使用 `Dio` 实例进行 GET 或者 POST 请求。例如,使用 GET 方法请求一个 JSON 数据:
```dart
Future<void> fetchAlbum() async {
final dio = Dio();
final response = await dio.get('https://jsonplaceholder.typicode.com/albums/1');
if (response.statusCode == 200) {
// 请求成功,处理响应数据
print(response.data);
} else {
// 请求失败,处理错误信息
print('请求失败,状态码为 ${response.statusCode}');
}
}
```
以上就是 Flutter 中进行网络请求的基本方法。需要注意的是,网络请求需要在异步环境下进行,因此需要使用 `async/await` 或者 `Future.then` 等方法处理异步操作。同时,还需要处理请求成功和请求失败的情况,以及错误信息的展示和处理。
阅读全文
相关推荐
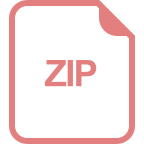
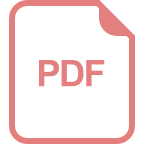

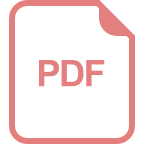
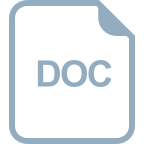
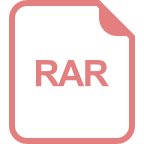
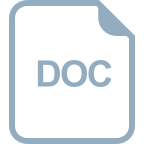
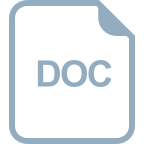
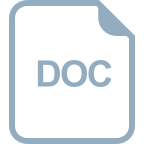
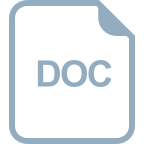
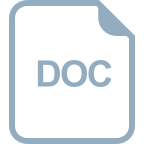
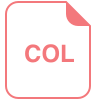
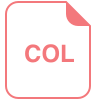
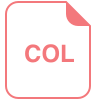

