进程调度算法模拟程序代码
时间: 2023-12-08 13:55:38 浏览: 40
以下是一个简单的进程调度算法模拟程序代码,使用了先来先服务(FCFS)和短作业优先(SJF)两种调度算法。程序使用 C 语言编写。
```c
#include <stdio.h>
struct process {
int pid; // 进程 ID
int arrival; // 到达时间
int burst; // 执行时间
int wait; // 等待时间
int turnaround; // 周转时间
};
void fcfs(struct process* p, int n);
void sjf(struct process* p, int n);
int main() {
struct process p[] = {
{1, 0, 8, 0, 0},
{2, 1, 4, 0, 0},
{3, 2, 9, 0, 0},
{4, 3, 5, 0, 0},
{5, 4, 2, 0, 0},
};
int n = sizeof(p) / sizeof(struct process);
fcfs(p, n);
sjf(p, n);
return 0;
}
void fcfs(struct process* p, int n) {
printf("FCFS Algorithm:\n");
int time = 0;
float avg_wait = 0, avg_turnaround = 0;
for (int i = 0; i < n; i++) {
if (time < p[i].arrival) {
time = p[i].arrival;
}
p[i].wait = time - p[i].arrival;
p[i].turnaround = p[i].burst + p[i].wait;
time += p[i].burst;
printf("P%d: wait=%d, turnaround=%d\n", p[i].pid, p[i].wait, p[i].turnaround);
avg_wait += p[i].wait;
avg_turnaround += p[i].turnaround;
}
avg_wait /= n;
avg_turnaround /= n;
printf("Average Wait Time: %.2f\n", avg_wait);
printf("Average Turnaround Time: %.2f\n", avg_turnaround);
}
void sjf(struct process* p, int n) {
printf("SJF Algorithm:\n");
int time = 0, done = 0;
float avg_wait = 0, avg_turnaround = 0;
while (done < n) {
int shortest = -1;
for (int i = 0; i < n; i++) {
if (p[i].arrival <= time && p[i].burst > 0) {
if (shortest == -1 || p[i].burst < p[shortest].burst) {
shortest = i;
}
}
}
if (shortest == -1) {
time++;
continue;
}
p[shortest].wait = time - p[shortest].arrival;
p[shortest].turnaround = p[shortest].burst + p[shortest].wait;
time += p[shortest].burst;
p[shortest].burst = 0;
done++;
printf("P%d: wait=%d, turnaround=%d\n", p[shortest].pid, p[shortest].wait, p[shortest].turnaround);
avg_wait += p[shortest].wait;
avg_turnaround += p[shortest].turnaround;
}
avg_wait /= n;
avg_turnaround /= n;
printf("Average Wait Time: %.2f\n", avg_wait);
printf("Average Turnaround Time: %.2f\n", avg_turnaround);
}
```
该程序使用了一个 `struct` 结构体来表示每个进程的信息,包括进程 ID、到达时间、执行时间、等待时间和周转时间。`fcfs()` 函数使用先来先服务算法计算等待时间和周转时间,并输出每个进程的信息和平均等待时间和平均周转时间。`sjf()` 函数使用短作业优先算法计算等待时间和周转时间,并输出每个进程的信息和平均等待时间和平均周转时间。程序中的进程信息是硬编码的,实际应用中应该从文件或用户输入中读取。
相关推荐
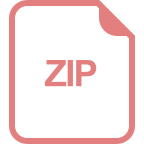
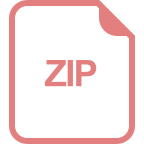
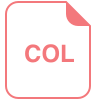
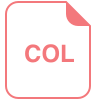
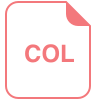
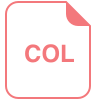
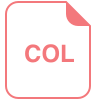









