java中print()方法写一个实例
时间: 2024-05-13 20:15:29 浏览: 93
下面是一个 Java 中使用 `print()` 方法的实例:
```java
public class PrintExample {
public static void main(String[] args) {
int number = 10;
double decimal = 3.14;
String name = "Java";
System.out.print("The number is: ");
System.out.print(number);
System.out.print("\nThe decimal is: ");
System.out.print(decimal);
System.out.print("\nThe name is: ");
System.out.print(name);
}
}
```
输出结果为:
```
The number is: 10
The decimal is: 3.14
The name is: Java
```
在这个例子中,我们定义了一个整型变量 `number`、一个浮点型变量 `decimal` 和一个字符串变量 `name`,然后使用 `print()` 方法输出这些变量的值。我们使用 `\n` 来换行输出。
相关问题
用Java写一个Student类,它有一个实例变量name,代表学生的名字,编写一个printName方法
public class Student {
private String name;
public Student(String name) {
this.name = name;
}
public void printName() {
System.out.println("Name: " + name);
}
}
用java中集合写几个实例
1. ArrayList实例:
```java
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
// create a new ArrayList
ArrayList<String> names = new ArrayList<>();
// add elements to the ArrayList
names.add("Alice");
names.add("Bob");
names.add("Charlie");
names.add("David");
// print the ArrayList
System.out.println(names);
// access an element of the ArrayList
System.out.println("The second element is " + names.get(1));
// remove an element from the ArrayList
names.remove(2);
// print the ArrayList again
System.out.println(names);
// get the size of the ArrayList
System.out.println("The size of the ArrayList is " + names.size());
}
}
```
2. HashSet实例:
```java
import java.util.HashSet;
public class HashSetExample {
public static void main(String[] args) {
// create a new HashSet
HashSet<String> names = new HashSet<>();
// add elements to the HashSet
names.add("Alice");
names.add("Bob");
names.add("Charlie");
names.add("David");
// print the HashSet
System.out.println(names);
// check if an element is in the HashSet
System.out.println("Is Bob in the HashSet? " + names.contains("Bob"));
// remove an element from the HashSet
names.remove("Charlie");
// print the HashSet again
System.out.println(names);
// get the size of the HashSet
System.out.println("The size of the HashSet is " + names.size());
}
}
```
3. HashMap实例:
```java
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
// create a new HashMap
HashMap<String, Integer> scores = new HashMap<>();
// add key-value pairs to the HashMap
scores.put("Alice", 90);
scores.put("Bob", 80);
scores.put("Charlie", 70);
scores.put("David", 60);
// print the HashMap
System.out.println(scores);
// access a value in the HashMap using the key
System.out.println("Alice's score is " + scores.get("Alice"));
// remove a key-value pair from the HashMap
scores.remove("Charlie");
// print the HashMap again
System.out.println(scores);
// get the size of the HashMap
System.out.println("The size of the HashMap is " + scores.size());
}
}
```
阅读全文
相关推荐

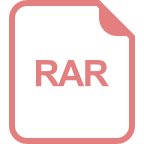
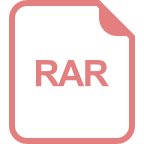
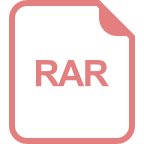









