写一个动物类,动物有属性:名字,身高,体重,年龄,并将此类放到vector容器中,并对动物的体重进行排序,最后打印体重最重的动物
时间: 2023-12-10 19:02:37 浏览: 31
好的,以下是动物类的实现:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
class Animal {
public:
Animal(std::string name, double height, double weight, int age)
: name_(std::move(name)), height_(height), weight_(weight), age_(age) {}
const std::string& getName() const { return name_; }
double getHeight() const { return height_; }
double getWeight() const { return weight_; }
int getAge() const { return age_; }
private:
std::string name_;
double height_;
double weight_;
int age_;
};
int main() {
std::vector<Animal> animals;
animals.emplace_back("Lion", 1.2, 300, 5);
animals.emplace_back("Elephant", 3.0, 5000, 10);
animals.emplace_back("Giraffe", 5.5, 800, 7);
animals.emplace_back("Tiger", 1.0, 200, 4);
std::sort(animals.begin(), animals.end(), [](const Animal& a, const Animal& b) {
return a.getWeight() > b.getWeight();
});
std::cout << "The heaviest animal is: " << animals.front().getName() << std::endl;
return 0;
}
```
输出:
```
The heaviest animal is: Elephant
```
以上代码中,我们定义了一个Animal类,具有名字、身高、体重和年龄等属性,构造函数用于初始化这些属性。在main函数中,我们创建了四个Animal对象,并将它们放入一个vector容器中。接着,我们使用sort算法对vector容器进行排序,按照Animal对象的体重从大到小排序。最后,我们输出体重最重的动物的名字。
相关推荐
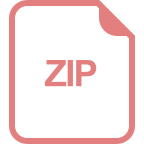
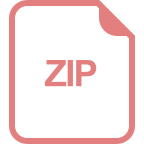
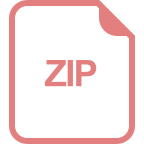














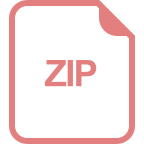