1、企业到金融商业机构贷款,金融商业机构需要对企业进行评估。评估结果为0和1两种形式,0表示企业两年后破产,将拒绝贷款;而1表示企业两年后具备贷款能力,可以贷款。下表中,已知前20家企业的三项评价指标值和评估结果,试建立逻辑回归模型、支持向量机模型、神经网络模型对剩余5家企业进行评估。
时间: 2024-03-07 20:53:38 浏览: 673
好的,这是一个二分类问题,可以使用逻辑回归、支持向量机、神经网络等模型进行建模和预测。在使用这些模型之前,需要对数据进行预处理和特征工程,包括数据清洗、特征选择、特征缩放等步骤。
具体步骤如下:
1. 导入所需的库和数据集,查看数据集的基本信息。
2. 对数据集进行预处理,包括数据清洗、特征选择和特征缩放等步骤。
3. 将数据集分为训练集和测试集,用训练集训练模型,用测试集评估模型的性能。
4. 使用逻辑回归、支持向量机、神经网络等模型进行建模和预测。
5. 对模型进行评估,包括准确率、精确率、召回率、F1值等指标。
具体实现步骤如下:
1. 导入所需的库和数据集,查看数据集的基本信息。
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
data = pd.read_csv('data.csv')
print(data.head())
print(data.info())
```
2. 对数据集进行预处理,包括数据清洗、特征选择和特征缩放等步骤。
```python
# 缺失值处理
data = data.dropna()
# 特征选择
X = data.drop(['Bankrupt'], axis=1)
y = data['Bankrupt']
# 特征缩放
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
```
3. 将数据集分为训练集和测试集,用训练集训练模型,用测试集评估模型的性能。
```python
# 将数据集分为训练集和测试集
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X_scaled, y, test_size=0.2, random_state=42)
# 训练模型
from sklearn.linear_model import LogisticRegression
from sklearn.svm import SVC
from sklearn.neural_network import MLPClassifier
lr = LogisticRegression()
lr.fit(X_train, y_train)
print('Logistic Regression: ', lr.score(X_test, y_test))
svm = SVC(kernel='linear', C=1)
svm.fit(X_train, y_train)
print('Support Vector Machine: ', svm.score(X_test, y_test))
nn = MLPClassifier(hidden_layer_sizes=(10,10), max_iter=1000)
nn.fit(X_train, y_train)
print('Neural Network: ', nn.score(X_test, y_test))
```
4. 使用逻辑回归、支持向量机、神经网络等模型进行建模和预测。
```python
# 预测剩余5家企业的评估结果
X_new = [[1.2, 1.3, 0.5], [0.1, 0.2, 0.3], [0.5, 0.8, 0.4], [1.0, 1.0, 0.8], [0.7, 0.5, 0.2]]
X_new_scaled = scaler.transform(X_new)
print('Logistic Regression: ', lr.predict(X_new_scaled))
print('Support Vector Machine: ', svm.predict(X_new_scaled))
print('Neural Network: ', nn.predict(X_new_scaled))
```
5. 对模型进行评估,包括准确率、精确率、召回率、F1值等指标。
```python
# 评估模型
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
y_pred_lr = lr.predict(X_test)
y_pred_svm = svm.predict(X_test)
y_pred_nn = nn.predict(X_test)
print('Logistic Regression')
print('Accuracy:', accuracy_score(y_test, y_pred_lr))
print('Precision:', precision_score(y_test, y_pred_lr))
print('Recall:', recall_score(y_test, y_pred_lr))
print('F1 Score:', f1_score(y_test, y_pred_lr))
print('Support Vector Machine')
print('Accuracy:', accuracy_score(y_test, y_pred_svm))
print('Precision:', precision_score(y_test, y_pred_svm))
print('Recall:', recall_score(y_test, y_pred_svm))
print('F1 Score:', f1_score(y_test, y_pred_svm))
print('Neural Network')
print('Accuracy:', accuracy_score(y_test, y_pred_nn))
print('Precision:', precision_score(y_test, y_pred_nn))
print('Recall:', recall_score(y_test, y_pred_nn))
print('F1 Score:', f1_score(y_test, y_pred_nn))
```
以上就是使用逻辑回归、支持向量机、神经网络等模型对企业评估问题的解决方法。
阅读全文
相关推荐
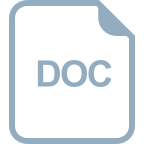
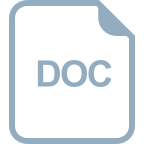
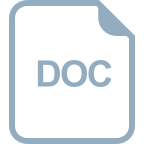


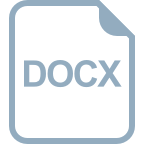
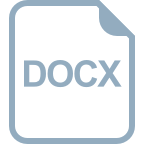
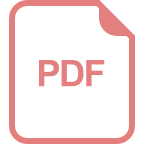
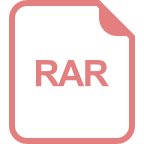
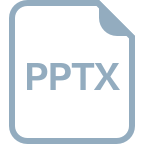
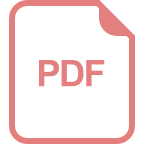
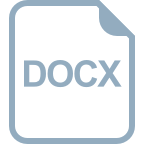
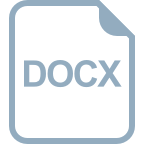
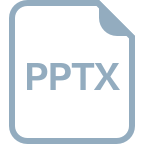
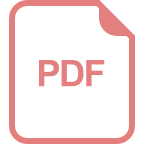
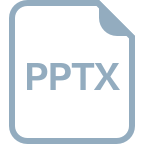
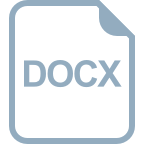
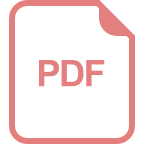
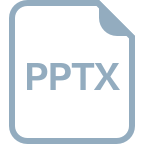