list<string>转换成多叉树结构代码
时间: 2023-11-10 11:04:23 浏览: 142
这里提供一种基于递归的实现方式,假设多叉树节点的定义如下:
```
class Node {
public:
string val;
vector<Node*> children;
};
```
其中,val表示节点的值,children表示子节点列表。
递归的实现思路如下:
1. 判断list是否为空,如果是则返回空指针。
2. 取出list的第一个元素作为根节点的值。
3. 创建一个新的节点,将根节点的值赋给该节点的val属性。
4. 遍历list中剩余的元素,将每个元素作为一个子树的根节点,递归处理该子树。
5. 将处理得到的子树添加到根节点的children列表中。
6. 返回根节点。
具体的代码实现如下:
```
Node* listToTree(list<string>& lst) {
if (lst.empty()) {
return nullptr;
}
string rootVal = lst.front();
lst.pop_front();
Node* root = new Node();
root->val = rootVal;
while (!lst.empty() && lst.front() != rootVal) {
Node* child = listToTree(lst);
if (child) {
root->children.emplace_back(child);
}
}
return root;
}
```
使用示例:
```
list<string> lst {"A", "B", "E", "F", "C", "G", "H", "D", "I", "J", "K"};
Node* root = listToTree(lst);
```
上述代码将会生成如下的多叉树结构:
```
A
/ / | \ \
B E F C G
/ \
H D
/ \
I J
/ \
K L
```
阅读全文
相关推荐
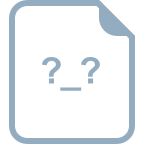
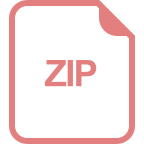
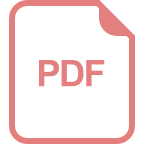


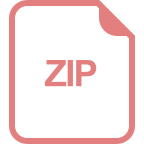
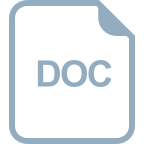
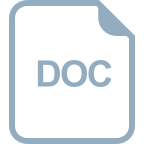
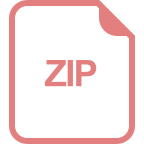
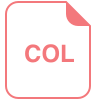
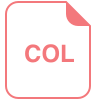
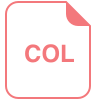
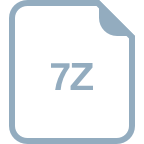
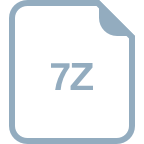
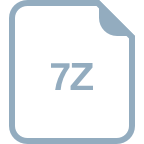
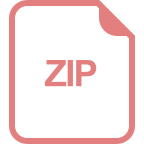
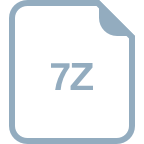
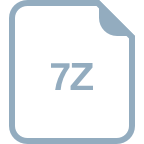
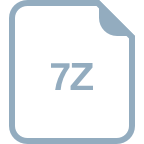