dilation = cv2.dilate(matrix.astype(np.uint8), kernel, iterations=1)中,iterations的值可以设置成多少
时间: 2024-04-04 14:29:13 浏览: 141
`iterations`参数指定对输入矩阵进行膨胀运算的次数。每次膨胀运算都会使白色区域扩张,因此可以通过增加`iterations`的值来使膨胀效果更加明显。
默认情况下,`iterations`参数的值为1,这意味着每个像素只会参与一次膨胀运算。如果将`iterations`参数设置为2或更高的值,可以使膨胀效果更加强烈。
需要注意的是,`iterations`参数的值过大可能会导致结果出现过度膨胀或者出现噪点等问题。因此,在实际应用中需要根据具体情况来调整`iterations`参数的值。
总之,`iterations`参数的值可以根据需要进行调整,常用的取值范围为1~10之间。
相关问题
程序运行提示OSError: cannot write mode F as BMP,修改gray = matrix * 255 # 进行膨胀运算 kernel = np.ones((3, 3), np.uint8) dilation = cv2.dilate(gray, kernel, iterations=1) # 显示膨胀后的图像 cv2.imshow('Dilation', dilation) img_1 = Image.fromarray(dilation * 255) img_1.save('dilation_high_1.bmp')
这个错误提示是由于你正在尝试将一个数据类型为 `float` 的数组转换为 BMP 文件格式,但是 BMP 格式不支持浮点数格式。因此,需要将数组中的值乘以 255,将其转换为 8 位无符号整数格式。以下是修改后的代码:
``` python
import cv2
import numpy as np
from PIL import Image
with open('point.txt', 'r') as f:
lines = f.readlines()
matrix = np.zeros((m, n))
for line in lines:
values = line.split()
i = int((float(values[0]) - col1_min) / avg_interval_dx)
j = int((float(values[1]) - col2_min) / (avg_interval_dy * 3))
matrix[i, j] = float(values[2])
# 将矩阵转换为灰度图像
gray = (matrix * 255).astype(np.uint8)
# 进行膨胀运算
kernel = np.ones((3, 3), np.uint8)
dilation = cv2.dilate(gray, kernel, iterations=1)
# 显示膨胀后的图像
cv2.imshow('Dilation', dilation)
# 将图像保存为 BMP 格式
img_1 = Image.fromarray(dilation)
img_1.save('dilation_high_1.bmp')
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这里,我们使用了 NumPy 库中的 `astype()` 函数,将矩阵中的浮点数值转换为 8 位无符号整数格式。然后,我们使用 PIL 库中的 `Image.fromarray()` 函数将 NumPy 数组转换为图像,并将其保存为 BMP 格式。
mport cv2 import numpy as np svm = cv2.ml.SVM_load("svm.xml") cap = cv2.VideoCapture(0) while True: ret, frame = cap.read() gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) _, thresh = cv2.threshold(gray, 120, 255, cv2.THRESH_BINARY_INV) kernel = np.ones((5,5), np.uint8) dilation = cv2.dilate(thresh, kernel, iterations=1) contours, hierarchy = cv2.findContours(dilation, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) for contour in contours: (x, y, w, h) = cv2.boundingRect(contour) if w < 5 or h < 5: continue cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2) roi = thresh[y:y + h, x:x + w] roi = cv2.resize(roi, (28, 28), interpolation=cv2.INTER_AREA) feature = np.reshape(roi, (1, 28 * 28)).astype(np.float32) result = svm.predict(feature)[1][0][0] cv2.putText(frame, str(int(result)), (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2) cv2.imshow("frame", frame) if cv2.waitKey(1) & 0xFF == ord('q'): break # 关闭摄像头 cap.release() cv2.destroyAllWindows()
这段代码使用OpenCV和SVM模型来实现实时数字识别。以下是代码的一些解释:
1. 导入OpenCV和numpy库,并加载SVM模型。
2. 打开本地摄像头,并在while循环中读取每一帧图像。
3. 将图像转换为灰度图像,并进行二值化和膨胀,以便更好地识别数字轮廓。
4. 使用OpenCV的轮廓检测功能,找到数字轮廓并提取出数字的特征。例如,数字的高度,宽度,以及数字与周围元素的相对位置。
5. 使用SVM模型对数字特征进行预测,并在原图像上用矩形框标出数字区域,并将识别结果显示在图像上。
6. 如果按下'q'键,则退出while循环。
7. 最后,释放摄像头,关闭所有窗口。
需要注意的是,这段代码中的数字识别准确率取决于训练集的大小和质量,以及所使用的机器学习算法的性能。在实际应用中,还需要考虑光线、角度、距离等因素对数字识别的影响。
阅读全文
相关推荐
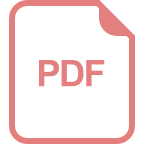
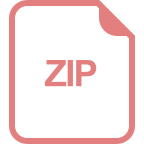
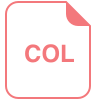
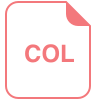




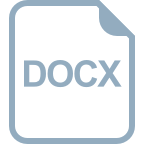
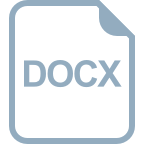
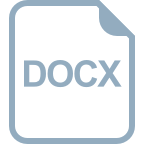
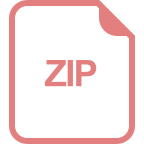