给所有代码加上注释
时间: 2023-07-03 14:17:26 浏览: 87
好的,以下是加上注释的代码:
```java
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class CarSalesStats {
// 定义Mapper类
public static class CarSalesMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1); // 定义一个常量1
private Text brand = new Text(); // 定义一个Text类型的变量
// 实现map函数
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(","); // 将输入的CSV文件中每行数据以逗号为分隔符进行分割
brand.set(fields[0]); // 将第一个字段(汽车品牌)作为键
context.write(brand, one); // 将值设为1,表示销售数量为1
}
}
// 定义Reducer类
public static class CarSalesReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
// 实现reduce函数
public void reduce(Text key, Iterable<IntWritable> values, Context context)
throws IOException, InterruptedException {
int sum = 0;
for (IntWritable value : values) {
sum += value.get(); // 对每个品牌的销售数量进行累加求和
}
context.write(key, new IntWritable(sum)); // 输出每个品牌的销售数量
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Car Sales Stats"); // 创建一个作业实例
job.setJarByClass(CarSalesStats.class); // 设置作业的主类
job.setMapperClass(CarSalesMapper.class); // 设置Mapper类
job.setReducerClass(CarSalesReducer.class); // 设置Reducer类
job.setOutputKeyClass(Text.class); // 设置输出的键类型
job.setOutputValueClass(IntWritable.class); // 设置输出的值类型
FileInputFormat.addInputPath(job, new Path(args[0])); // 设置输入路径
FileOutputFormat.setOutputPath(job, new Path(args[1])); // 设置输出路径
System.exit(job.waitForCompletion(true) ? 0 : 1); // 启动作业并等待完成
}
}
```
希望这样更容易理解了。
阅读全文
相关推荐
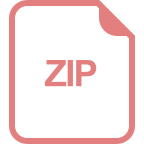














