python 画条带图
时间: 2023-08-13 07:03:39 浏览: 143
### 回答1:
好的,我可以帮你解决这个问题。你可以使用Python中的Matplotlib库进行绘制。你可以通过以下代码来绘制一条带图:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成随机数
x = np.arange(0, 10, 0.1)
y = np.sin(x)
# 绘制条带图
plt.fill_between(x, y, 0, where=(y > 0), interpolate=True, color='green', alpha=0.3)
plt.fill_between(x, y, 0, where=(y < 0), interpolate=True, color='red', alpha=0.3)
# 设置图表标题
plt.title("Strip Plot")
# 显示图表
plt.show()
```
这段代码将生成一条带图,其中正数为绿色,负数为红色。你可以使用Matplotlib库的其他功能来添加其他特殊效果,以使你的图表更加出色。希望这个答案可以帮助到你!
### 回答2:
python可以使用matplotlib库来画条带图。下面是一个简单的例子:
```python
import matplotlib.pyplot as plt
# 定义数据
labels = ['A', 'B', 'C', 'D']
values = [20, 35, 30, 15]
# 画条带图
plt.bar(labels, values, color=['red', 'green', 'blue', 'yellow'])
# 设置图标题和轴标签
plt.title('Bar Chart')
plt.xlabel('Labels')
plt.ylabel('Values')
# 显示图形
plt.show()
```
这个例子中,我们首先定义了标签(labels)和对应的数值(values),然后使用`plt.bar`函数来画条带图。通过设置`color`参数,我们可以为每个条带指定不同的颜色。接着,我们使用`plt.title`、`plt.xlabel`和`plt.ylabel`函数来设置图的标题和轴标签。最后,使用`plt.show`函数显示图形。
当运行这段代码时,将会显示一个有四个条带的条带图,每个条带代表一个类别,高度表示其对应的数值。可以根据需要自定义标签、数值和颜色来画出不同的条带图。
### 回答3:
Python是一种非常强大的编程语言,用它可以实现各种绘图功能。要画一幅条带图,我们可以借助Python的绘图库matplotlib来实现。
首先,我们需要导入matplotlib库和numpy库(用于生成数据),并创建一个绘图窗口。
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
接下来,我们可以使用numpy的arange函数生成一个数组,作为x轴的数据。
x = np.arange(0, 10, 0.1)
然后,我们定义一个函数来生成y轴的数据,例如使用sin函数生成一个周期为2π的正弦曲线。
def generate_y(x):
return np.sin(x)
将x轴数据传入函数中,生成对应的y轴数据。
y = generate_y(x)
最后,我们可以使用plot函数绘制出条带图。
ax.plot(x, y)
为了美化图形,我们可以添加标题、坐标轴标签和图例等。
ax.set_title("Striped Graph")
ax.set_xlabel("x")
ax.set_ylabel("y")
ax.legend(["sin(x)"])
最后,我们调用show函数显示图形。
plt.show()
通过以上步骤,我们就可以使用Python绘制出一幅条带图了。如果需要更多样式的条带图,可以通过修改x轴和y轴的数据、函数等来实现。
阅读全文
相关推荐
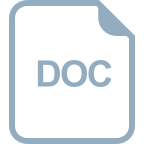
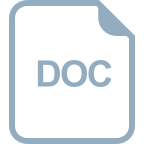
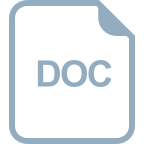















