利用栈将十进制整数转化为二进制数的c语言代码
时间: 2023-05-31 10:20:59 浏览: 201
### 回答1:
以下是利用栈将十进制整数转化为二进制数的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 100
typedef struct {
int top;
int data[STACK_SIZE];
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -1;
}
int isFull(Stack *s) {
return s->top == STACK_SIZE - 1;
}
void push(Stack *s, int x) {
if (isFull(s)) {
printf("Stack overflow\n");
exit(1);
}
s->data[++s->top] = x;
}
int pop(Stack *s) {
if (isEmpty(s)) {
printf("Stack underflow\n");
exit(1);
}
return s->data[s->top--];
}
void decimalToBinary(int n) {
Stack s;
initStack(&s);
while (n > ) {
push(&s, n % 2);
n /= 2;
}
while (!isEmpty(&s)) {
printf("%d", pop(&s));
}
}
int main() {
int n;
printf("Enter a decimal number: ");
scanf("%d", &n);
printf("Binary equivalent: ");
decimalToBinary(n);
printf("\n");
return ;
}
```
### 回答2:
要用栈将十进制整数转化为二进制数的c语言代码,首先需要明白十进制和二进制之间的转换方式。我们知道,二进制数是由0和1组成的数字系统,而十进制数是由0到9这10个数字组成的数字系统。将一个十进制数转化为二进制数的方法是不断将该数除以2,把余数加到一个栈里面,最后把栈里的余数依次取出即可得到该数的二进制表示。
下面是利用栈将十进制整数转化为二进制数的c语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define SIZE 100
// 栈的定义
typedef struct stack{
int data[SIZE];
int top;
} Stack;
// 初始化栈
void init(Stack *s){
s->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack *s){
return s->top == -1 ? 1 : 0;
}
// 判断栈是否已满
int isFull(Stack *s){
return s->top == SIZE - 1 ? 1 : 0;
}
// 添加元素
void push(Stack *s, int num){
if(isFull(s)){
printf("栈已满,无法入栈");
return;
}else{
s->top++;
s->data[s->top] = num;
}
}
// 删除元素
int pop(Stack *s){
if(isEmpty(s)){
printf("栈已空,无法出栈");
return -1;
}else{
int num = s->data[s->top];
s->top--;
return num;
}
}
// 采用栈的方式转化为二进制数
void decimalToBinary(int num){
Stack s; // 建立栈
int rem;
init(&s); // 初始化栈
while(num != 0){ // 不断将十进制数除以2,余数压入栈
rem = num % 2;
push(&s, rem);
num = num / 2;
}
printf("转换后的二进制数为:");
while(!isEmpty(&s)){ // 将栈中的余数依次取出,得到二进制数
printf("%d", pop(&s));
}
}
int main(){
int num;
printf("请输入一个十进制整数:");
scanf("%d", &num);
decimalToBinary(num); // 转换为二进制数
return 0;
}
```
上述代码中,我们采用了栈的方式来转化为二进制数,利用while循环不断将十进制数除以2,余数压入栈中;然后再通过while循环将栈中的余数依次取出,得到二进制数。其中,init函数用于初始化栈,push函数用于向栈中添加元素,pop函数用于删除栈顶元素,isEmpty函数用于判断栈是否为空,isFull函数用于判断栈是否已满。请注意,栈的最大容量被定义为100,如果栈已满或为空,则无法继续压入元素或弹出元素。
### 回答3:
十进制整数转换为二进制数可以使用栈的数据结构来实现。代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 256
// 栈结构体
typedef struct Stack {
int data[STACK_SIZE];
int top;
} Stack;
// 初始化栈
void InitStack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int IsEmpty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int IsFull(Stack *s) {
return s->top == STACK_SIZE - 1;
}
// 入栈操作
void PushStack(Stack *s, int x) {
if (IsFull(s)) {
printf("Stack is Full!\n");
return;
}
s->data[++s->top] = x;
}
// 出栈操作
int PopStack(Stack *s) {
if (IsEmpty(s)) {
printf("Stack is Empty!\n");
exit(1);
}
return s->data[s->top--];
}
// 十进制转二进制
void DecimalToBinary(int n) {
Stack s;
InitStack(&s);
while (n) {
PushStack(&s, n % 2);
n /= 2;
}
while (!IsEmpty(&s)) {
printf("%d", PopStack(&s));
}
}
int main() {
int n;
printf("Please input a decimal integer: ");
scanf("%d", &n);
DecimalToBinary(n);
printf("\n");
return 0;
}
```
上述代码中,首先定义了一个栈结构体,并实现了栈的初始化、入栈、出栈等基本操作。接着,使用栈实现了十进制整数转二进制数的功能,具体实现方式为:将待转换的十进制数不断除以2,将余数依次入栈,最后依次出栈输出即可。通过该程序可以将十进制整数转化为二进制数。
阅读全文
相关推荐





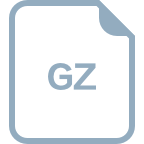









