php base64转图片
时间: 2023-07-18 20:39:51 浏览: 43
可以使用以下代码将Base64编码的字符串转换为图片文件:
```php
// 获取Base64编码的字符串
$base64_string = "data:image/png;base64,iVBORw0KG...";
// 将字符串转换为文件流
$decoded_string = base64_decode(preg_replace('#^data:image/\w+;base64,#i', '', $base64_string));
// 保存文件
$file_name = "image.png";
$file = fopen($file_name, "wb");
fwrite($file, $decoded_string);
fclose($file);
```
在上面的代码中,`$base64_string`是Base64编码的字符串,`$file_name`是要保存的文件名。在将字符串转换为文件流之前,需要使用正则表达式去掉Base64字符串中的数据头部分。最后,将文件流写入到指定的文件中即可。
相关问题
php 转 图片base64
PHP可以通过读取图片文件并将其转换为base64编码格式来实现将图片以base64显示的功能。具体实现方法如下:
1. 读取图片文件并获取图片信息,可以使用getimagesize()函数。
2. 将图片文件内容转换为base64编码格式,可以使用file_get_contents()函数和base64_encode()函数。
3. 将base64编码格式的图片内容嵌入到HTML代码中,可以使用<img>标签,并将src属性设置为"data:image/png;base64,..."的形式,其中"data:image/png;base64,"是固定的前缀,后面跟着base64编码格式的图片内容。
以下是一个示例代码:
```
$image_file = './example.jpg';
$image_info = getimagesize($image_file);
$image_data = file_get_contents($image_file);
$base64_image = 'data:' . $image_info['mime'] . ';base64,' . base64_encode($image_data);
echo '<img src="' . $base64_image . '">';
```
这段代码将读取名为example.jpg的图片文件,并将其转换为base64编码格式,最后将其嵌入到HTML代码中以显示图片。
PHP图片转base64
PHP图片转base64是一种将图片文件转换为base64编码的方法。这种方法可以将图片文件转换为一段文本,方便在网络传输中使用。通常情况下,我们可以使用PHP的getimagesize函数获取图片的信息,然后使用fread函数读取图片文件的内容,最后使用base64_encode函数将图片内容进行编码。下面是一个示例代码:
```
function base64EncodeImage($image_file) {
$base64_image = '';
$image_info = getimagesize($image_file);
$image_data = fread(fopen($image_file, 'r'), filesize($image_file));
$base64_image = chunk_split(base64_encode($image_data));
return $base64_image;
}
```
这个函数接受一个图片文件路径作为参数,返回一个base64编码的字符串。在使用时,只需要调用这个函数并传入图片文件路径即可。
相关推荐
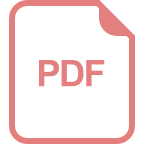











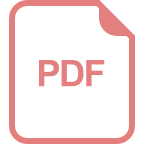