两类感知器参数学习算法的简单实现输出实例
时间: 2023-08-09 22:11:47 浏览: 47
好的,我可以为您提供两类感知器参数学习算法的简单实现输出实例。这两个算法分别是 Rosenblatt 感知器算法和 Widrow-Hoff 算法。
1. Rosenblatt 感知器算法实现输出实例:
```python
import numpy as np
class Perceptron:
def __init__(self, input_size, lr=1, epochs=100):
self.W = np.zeros(input_size+1)
self.epochs = epochs
self.lr = lr
def activation_fn(self, x):
return 1 if x >= 0 else -1
def predict(self, x):
x = np.insert(x, 0, 1)
z = self.W.T.dot(x)
a = self.activation_fn(z)
return a
def fit(self, X, d):
for epoch in range(self.epochs):
for i in range(d.shape[0]):
x = X[i]
y = self.predict(x)
e = d[i] - y
x = np.insert(x, 0, 1)
self.W = self.W + self.lr * e * x
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
d = np.array([-1, -1, -1, 1])
perceptron = Perceptron(input_size=2)
perceptron.fit(X, d)
print(perceptron.W)
```
2. Widrow-Hoff 算法实现输出实例:
```python
import numpy as np
class WidrowHoff:
def __init__(self, input_size, lr=0.1, epochs=100):
self.W = np.zeros(input_size+1)
self.epochs = epochs
self.lr = lr
def activation_fn(self, x):
return x
def predict(self, x):
x = np.insert(x, 0, 1)
z = self.W.T.dot(x)
a = self.activation_fn(z)
return a
def fit(self, X, d):
for epoch in range(self.epochs):
for i in range(d.shape[0]):
x = X[i]
y = self.predict(x)
e = d[i] - y
x = np.insert(x, 0, 1)
self.W = self.W + self.lr * e * x * y
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
d = np.array([-1, -1, -1, 1])
widrow_hoff = WidrowHoff(input_size=2)
widrow_hoff.fit(X, d)
print(widrow_hoff.W)
```
希望这些输出实例能够帮助您更好地理解感知器算法,如果您有任何疑问,请随时问我。
相关推荐









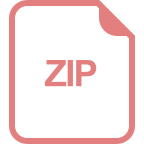
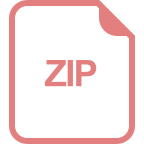
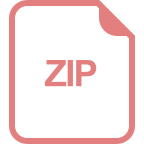
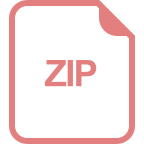
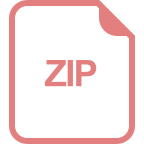
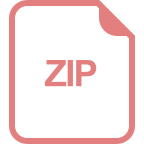
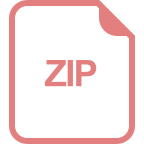