感知算法的设计与实现,对对鸢尾花种类的识别python代码
时间: 2023-12-12 07:02:34 浏览: 62
感知算法是一种简单的分类算法,适用于二分类问题。下面给出用感知算法实现对鸢尾花种类的识别的Python代码。
首先,导入必要的库:
```python
import numpy as np
import pandas as pd
from sklearn.datasets import load_iris
```
然后,加载鸢尾花数据集,并将其转化为DataFrame格式:
```python
iris = load_iris()
df = pd.DataFrame(data=iris.data, columns=iris.feature_names)
df['label'] = iris.target
df.columns = ['sepal length', 'sepal width', 'petal length', 'petal width', 'label']
df.head()
```
接着,将数据集划分为训练集和测试集:
```python
data = np.array(df.iloc[:100, [0, 1, -1]]) # 取前100个样本,两个特征和一个标签
X, y = data[:, :-1], data[:, -1]
y = np.array([1 if i == 1 else -1 for i in y]) # 将标签转化为 +1/-1 的形式
train_data, train_label = X[:40], y[:40]
test_data, test_label = X[40:], y[40:]
```
定义感知算法的类:
```python
class Perceptron:
def __init__(self):
self.w = np.zeros(train_data.shape[1])
self.b = 0
self.lr = 0.1
def sign(self, x, w, b):
y = np.dot(x, w) + b
return y
def fit(self, X_train, y_train):
is_wrong = False
while not is_wrong:
wrong_count = 0
for i in range(len(X_train)):
X = X_train[i]
y = y_train[i]
if y * self.sign(X, self.w, self.b) <= 0:
self.w = self.w + self.lr * np.dot(y, X)
self.b = self.b + self.lr * y
wrong_count += 1
if wrong_count == 0:
is_wrong = True
return 'Perceptron Model!'
def score(self, X_test, y_test):
right_count = 0
for i in range(len(X_test)):
X = X_test[i]
y = y_test[i]
if y * self.sign(X, self.w, self.b) > 0:
right_count += 1
return right_count / len(X_test)
```
最后,创建一个感知器实例,调用 `fit` 函数进行训练,调用 `score` 函数进行测试:
```python
perceptron = Perceptron()
perceptron.fit(train_data, train_label)
print(perceptron.score(test_data, test_label))
```
输出的结果为测试集上的准确率。
阅读全文
相关推荐
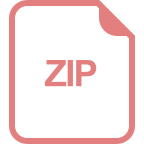
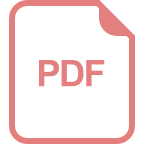

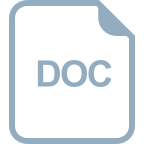
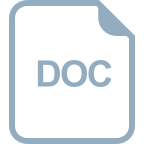
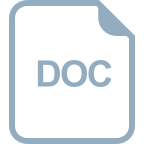
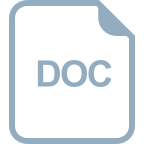
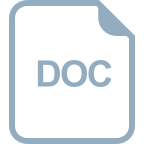
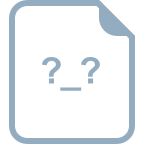
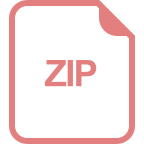
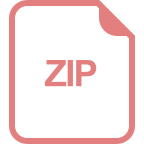
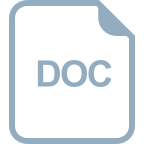
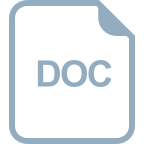
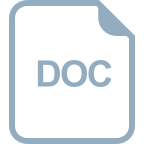
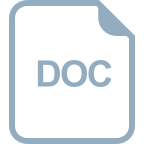
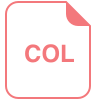
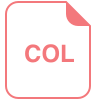
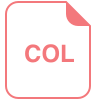