const Jimp = require('jimp'); const _ = require('lodash'); class parse_bg_captcha { // 初始化方法 constructor () { // 读取图片 this.imgPromise = Jimp.read("./bg.png"); // 还原后的背景图 this.newImg = new Jimp(260, 160); // 图片还原顺序, 定值 this.Ge = [39, 38, 48, 49, 41, 40, 46, 47, 35, 34, 50, 51, 33, 32, 28, 29, 27, 26, 36, 37, 31, 30, 44, 45, 43, 42, 12, 13, 23, 22, 14, 15, 21, 20, 8, 9, 25, 24, 6, 7, 3, 2, 0, 1, 11, 10, 4, 5, 19, 18, 16, 17]; this.w_sep = 10; this.h_sep = 80; } // 循环合成图片 async loop_montage_image (imgPath, imShow = false, savePath = null) { const img = await this.imgPromise; // 并行处理裁剪和拼接操作 const promises = this.Ge.map(idx => { const x = idx % 26 * 12 + 1; const y = idx > 25 ? this.h_sep : 0; const imgCut = _.cloneDeep(img).crop(x, y, this.w_sep, this.h_sep); const newX = idx % 26 * 10; const newY = idx > 25 ? this.h_sep : 0; return this.newImg.blitAsync(imgCut, newX, newY); }); await Promise.all(promises); if (imShow) { this.newImg.write('output.jpg'); } if (savePath !== null) { await this.newImg.writeAsync(savePath); } } // run方法 run (imgPath, imShow = false, savePath = null) { this.loop_montage_image(imgPath, imShow, savePath); } } if (__filename === process.mainModule.filename) { console.log("开始"); const c = new parse_bg_captcha(); c.run('./bg.png', true, 'bg.jpg'); } 这段代码帮我优化一下
时间: 2023-12-22 19:05:15 浏览: 114
这段代码已经很不错了,但是可以稍微进行一些优化和改进。以下是一些可能有用的建议:
1. 使用 ES6 的 class 语法来定义类,这样代码更加清晰易读。
2. 将图片读取操作放到 constructor 中,避免每次都重新读取图片。
3. 使用 Jimp 的 `clone()` 方法来避免使用 lodash 的 `cloneDeep()` 方法。
4. 将参数的默认值设为 null,而不是 false,这样可以更好地表示参数是否提供。
5. 将循环合成图片的方法命名为 `montageImage()`,更能描述其功能。
综上所述,以下是经过优化的代码:
```
const Jimp = require('jimp');
class ParseBgCaptcha {
constructor() {
// 读取图片
this.img = null;
Jimp.read('./bg.png').then((img) => {
this.img = img;
}).catch((err) => {
console.error(`读取图片失败: ${err}`);
});
// 还原后的背景图
this.newImg = new Jimp(260, 160);
// 图片还原顺序, 定值
this.Ge = [39, 38, 48, 49, 41, 40, 46, 47, 35, 34, 50, 51, 33, 32, 28, 29, 27, 26, 36, 37, 31, 30, 44, 45, 43, 42, 12, 13, 23, 22, 14, 15, 21, 20, 8, 9, 25, 24, 6, 7, 3, 2, 0, 1, 11, 10, 4, 5, 19, 18, 16, 17];
this.w_sep = 10;
this.h_sep = 80;
}
async montageImage(imgPath, imShow = null, savePath = null) {
const promises = this.Ge.map((idx) => {
const x = idx % 26 * 12 + 1;
const y = idx > 25 ? this.h_sep : 0;
const imgCut = this.img.clone().crop(x, y, this.w_sep, this.h_sep);
const newX = idx % 26 * 10;
const newY = idx > 25 ? this.h_sep : 0;
return this.newImg.blitAsync(imgCut, newX, newY);
});
await Promise.all(promises);
if (imShow) {
this.newImg.write('output.jpg');
}
if (savePath !== null) {
await this.newImg.writeAsync(savePath);
}
}
run(imgPath, imShow = null, savePath = null) {
this.montageImage(imgPath, imShow, savePath);
}
}
if (require.main === module) {
console.log('开始');
const c = new ParseBgCaptcha();
c.run('./bg.png', true, 'bg.jpg');
}
```
注意:在这个版本中,我们将默认值设为 null,然后在代码中检查是否提供参数。这样可以更好地检查参数是否存在,而不是只检查参数是否为真。
阅读全文
相关推荐
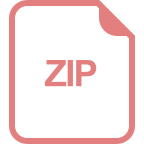
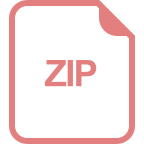
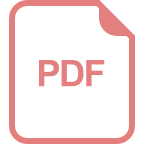

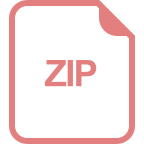
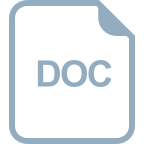
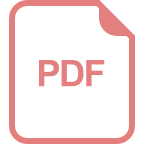
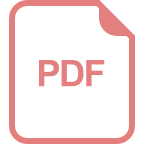
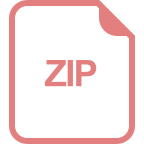
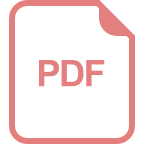
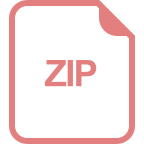
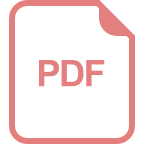
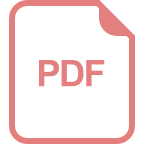
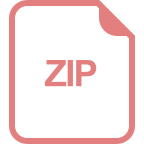
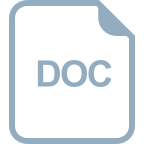
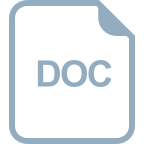
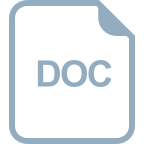
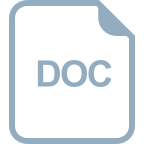