用一个结构体储存学生信息,在用链表访问学生结构体
时间: 2023-04-23 10:05:46 浏览: 93
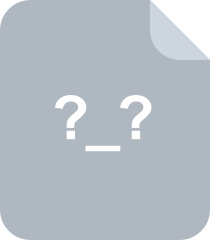
学生信息建立采用链表结构
可以定义一个包含学生信息的结构体,例如:
```
struct Student {
int id;
char name[20];
int age;
float score;
};
```
然后可以定义一个链表节点结构体,包含指向下一个节点的指针和一个学生信息结构体的指针,例如:
```
struct ListNode {
struct Student* data;
struct ListNode* next;
};
```
接下来可以定义一个链表结构体,包含指向链表头节点和尾节点的指针,例如:
```
struct LinkedList {
struct ListNode* head;
struct ListNode* tail;
};
```
最后可以实现链表的基本操作,例如创建链表、添加节点、删除节点、遍历节点等。具体实现可以参考以下代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
int id;
char name[20];
int age;
float score;
};
struct ListNode {
struct Student* data;
struct ListNode* next;
};
struct LinkedList {
struct ListNode* head;
struct ListNode* tail;
};
struct Student* createStudent(int id, char* name, int age, float score) {
struct Student* student = (struct Student*)malloc(sizeof(struct Student));
student->id = id;
strcpy(student->name, name);
student->age = age;
student->score = score;
return student;
}
struct ListNode* createListNode(struct Student* student) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->data = student;
node->next = NULL;
return node;
}
struct LinkedList* createLinkedList() {
struct LinkedList* list = (struct LinkedList*)malloc(sizeof(struct LinkedList));
list->head = NULL;
list->tail = NULL;
return list;
}
void addListNode(struct LinkedList* list, struct ListNode* node) {
if (list->head == NULL) {
list->head = node;
list->tail = node;
} else {
list->tail->next = node;
list->tail = node;
}
}
void removeListNode(struct LinkedList* list, struct ListNode* node) {
if (list->head == node) {
list->head = node->next;
} else {
struct ListNode* prev = list->head;
while (prev->next != node) {
prev = prev->next;
}
prev->next = node->next;
}
if (list->tail == node) {
list->tail = NULL;
}
free(node->data);
free(node);
}
void traverseLinkedList(struct LinkedList* list) {
struct ListNode* node = list->head;
while (node != NULL) {
printf("id: %d, name: %s, age: %d, score: %.2f\n", node->data->id, node->data->name, node->data->age, node->data->score);
node = node->next;
}
}
int main() {
struct LinkedList* list = createLinkedList();
struct Student* student1 = createStudent(1, "Tom", 18, 90.5);
struct Student* student2 = createStudent(2, "Jerry", 19, 85.0);
struct Student* student3 = createStudent(3, "Alice", 20, 95.0);
struct ListNode* node1 = createListNode(student1);
struct ListNode* node2 = createListNode(student2);
struct ListNode* node3 = createListNode(student3);
addListNode(list, node1);
addListNode(list, node2);
addListNode(list, node3);
traverseLinkedList(list);
removeListNode(list, node2);
traverseLinkedList(list);
return 0;
}
```
阅读全文
相关推荐
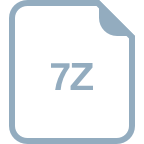
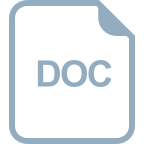




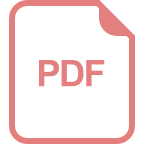
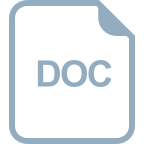
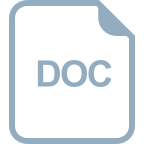





