使用Adaboost实现Iris分类,使用梯度提升树GBDT实现Boston房价预测(可以直接调函数)
时间: 2024-01-27 15:06:05 浏览: 98
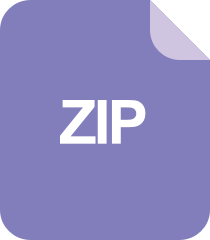
adaboost集成算法,可用来做房价的预测,matlab源码.zip
使用Adaboost实现Iris分类的示例代码如下:
```python
from sklearn.datasets import load_iris
from sklearn.ensemble import AdaBoostClassifier
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
# 加载数据
iris = load_iris()
X = iris.data
y = iris.target
# 切分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义分类器
dtc = DecisionTreeClassifier(max_depth=1)
# 定义Adaboost分类器,使用决策树作为基分类器
abc = AdaBoostClassifier(base_estimator=dtc, n_estimators=50)
# 训练模型
abc.fit(X_train, y_train)
# 预测
y_pred = abc.predict(X_test)
# 计算准确率
accuracy = (y_pred == y_test).sum() / len(y_test)
print("Accuracy:", accuracy)
```
使用梯度提升树GBDT实现Boston房价预测的示例代码如下:
```python
from sklearn.datasets import load_boston
from sklearn.ensemble import GradientBoostingRegressor
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# 加载数据
boston = load_boston()
X = boston.data
y = boston.target
# 切分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义回归器
gbr = GradientBoostingRegressor(n_estimators=100, learning_rate=0.1, max_depth=3)
# 训练模型
gbr.fit(X_train, y_train)
# 预测
y_pred = gbr.predict(X_test)
# 计算均方误差
mse = mean_squared_error(y_test, y_pred)
print("MSE:", mse)
```
阅读全文
相关推荐
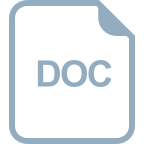
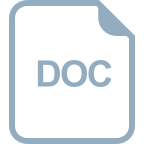



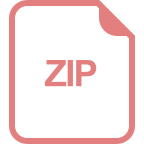
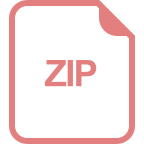
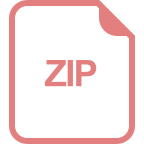
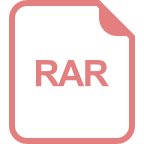
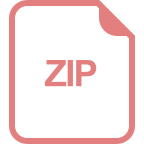
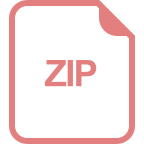
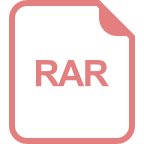
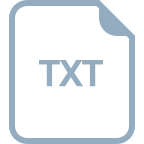
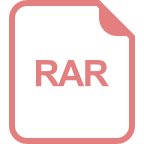
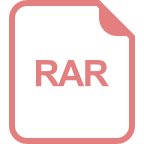
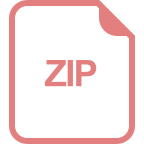