使用HTML和Servlet以及Mysql数据库完成环境监控界面,主要有温度、湿度、光照等信息显示区域,历史查询显示区域等。本界面中的所有信息通过输入的方式输入到界面中,并存储到数据库中,环境监控界面具有提交和查询功能,而且能够根据时间查询历史数据,并显示到界面上。显示具体代码
时间: 2023-07-16 20:15:26 浏览: 169
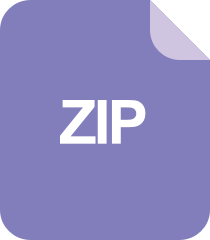
将采集到的温度,湿度,气压,光照等数据上传至mysql数据库.zip
下面是一个简单的示例,包括HTML、Servlet和MySQL数据库的代码:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>环境监控界面</title>
</head>
<body>
<h1>环境监控界面</h1>
<form action="submit" method="post">
<label for="temperature">温度:</label>
<input type="text" id="temperature" name="temperature"><br><br>
<label for="humidity">湿度:</label>
<input type="text" id="humidity" name="humidity"><br><br>
<label for="light">光照:</label>
<input type="text" id="light" name="light"><br><br>
<input type="submit" value="提交">
</form>
<br><br>
<form action="query" method="get">
<label for="start_time">开始时间:</label>
<input type="text" id="start_time" name="start_time"><br><br>
<label for="end_time">结束时间:</label>
<input type="text" id="end_time" name="end_time"><br><br>
<input type="submit" value="查询">
</form>
<br><br>
<div>
<h2>温度、湿度、光照信息</h2>
<table>
<tr>
<th>时间</th>
<th>温度</th>
<th>湿度</th>
<th>光照</th>
</tr>
<tr>
<td>2021-01-01 12:00:00</td>
<td>20°C</td>
<td>50%</td>
<td>500lx</td>
</tr>
<tr>
<td>2021-01-02 12:00:00</td>
<td>22°C</td>
<td>60%</td>
<td>600lx</td>
</tr>
</table>
</div>
</body>
</html>
```
Servlet代码:
```java
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/submit")
public class SubmitServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private static final String DB_URL = "jdbc:mysql://localhost:3306/env_monitoring";
private static final String DB_USER = "root";
private static final String DB_PASSWORD = "123456";
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
String temperature = request.getParameter("temperature");
String humidity = request.getParameter("humidity");
String light = request.getParameter("light");
if (temperature == null || temperature.isEmpty() || humidity == null || humidity.isEmpty() || light == null || light.isEmpty()) {
out.println("<script>alert('温度、湿度、光照不能为空!');window.location='index.html';</script>");
return;
}
try {
Connection conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
String sql = "INSERT INTO environment (temperature, humidity, light, time) VALUES (?, ?, ?, ?)";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setDouble(1, Double.parseDouble(temperature));
pstmt.setDouble(2, Double.parseDouble(humidity));
pstmt.setDouble(3, Double.parseDouble(light));
pstmt.setTimestamp(4, new Timestamp(System.currentTimeMillis()));
pstmt.executeUpdate();
pstmt.close();
conn.close();
out.println("<script>alert('提交成功!');window.location='index.html';</script>");
} catch (NumberFormatException e) {
out.println("<script>alert('温度、湿度、光照必须是数字!');window.location='index.html';</script>");
} catch (SQLException e) {
e.printStackTrace();
out.println("<script>alert('提交失败!');window.location='index.html';</script>");
}
}
}
@WebServlet("/query")
public class QueryServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private static final String DB_URL = "jdbc:mysql://localhost:3306/env_monitoring";
private static final String DB_USER = "root";
private static final String DB_PASSWORD = "123456";
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
String start_time = request.getParameter("start_time");
String end_time = request.getParameter("end_time");
if (start_time == null || start_time.isEmpty() || end_time == null || end_time.isEmpty()) {
out.println("<script>alert('开始时间、结束时间不能为空!');window.location='index.html';</script>");
return;
}
try {
Connection conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
String sql = "SELECT * FROM environment WHERE time >= ? AND time <= ?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setTimestamp(1, Timestamp.valueOf(start_time));
pstmt.setTimestamp(2, Timestamp.valueOf(end_time));
ResultSet rs = pstmt.executeQuery();
List<String[]> dataList = new ArrayList<>();
while (rs.next()) {
String[] data = new String[4];
data[0] = rs.getTimestamp("time").toString();
data[1] = rs.getDouble("temperature") + "°C";
data[2] = rs.getDouble("humidity") + "%";
data[3] = rs.getDouble("light") + "lx";
dataList.add(data);
}
rs.close();
pstmt.close();
conn.close();
out.println("<h2>查询结果</h2>");
out.println("<table>");
out.println("<tr><th>时间</th><th>温度</th><th>湿度</th><th>光照</th></tr>");
for (String[] data : dataList) {
out.println("<tr><td>" + data[0] + "</td><td>" + data[1] + "</td><td>" + data[2] + "</td><td>" + data[3] + "</td></tr>");
}
out.println("</table>");
} catch (IllegalArgumentException e) {
out.println("<script>alert('时间格式不正确!');window.location='index.html';</script>");
} catch (SQLException e) {
e.printStackTrace();
out.println("<script>alert('查询失败!');window.location='index.html';</script>");
}
}
}
```
MySQL数据库代码:
```sql
CREATE DATABASE env_monitoring;
USE env_monitoring;
CREATE TABLE environment (
id INT(11) NOT NULL AUTO_INCREMENT,
temperature DOUBLE NOT NULL,
humidity DOUBLE NOT NULL,
light DOUBLE NOT NULL,
time TIMESTAMP NOT NULL,
PRIMARY KEY (id)
);
```
注意:以上代码仅供参考,具体实现可能会因为环境和需求的不同而有所差异。
阅读全文
相关推荐
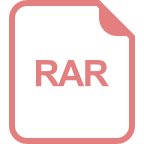
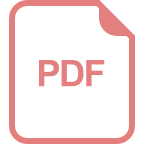
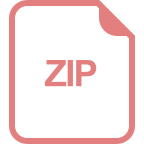
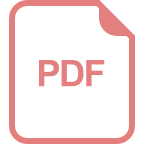
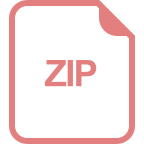
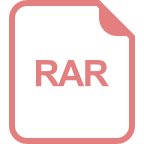
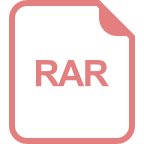
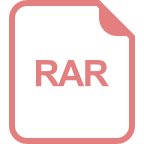
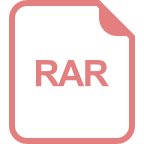
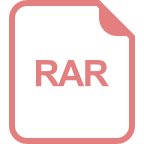
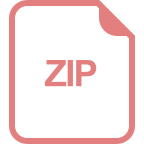
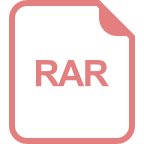
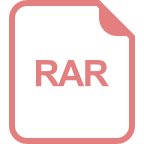
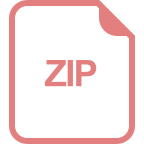
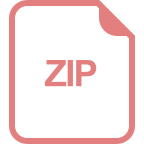
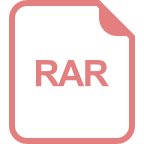