c 语言计算char* 字符串占用字节数完整代码示例
时间: 2023-08-12 11:03:58 浏览: 160
以下是一个计算 `char*` 字符串占用字节数的示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char *str = "Hello, world!";
int size = strlen(str) + 1; // 加 1 是为了包含字符串结尾的 NULL 字符
int byte_num = size * sizeof(char); // 计算占用字节数
printf("The size of the string is %d bytes.\n", byte_num);
return 0;
}
```
代码中,首先定义了一个 `char*` 类型的字符串 `str`,然后使用 `strlen()` 函数计算字符串的长度(不包括结尾的 NULL 字符),再加上 1 后即为字符串占用的总字节数。最后使用 `sizeof()` 函数计算 `char` 类型的大小,乘以字符串占用的字节数即为最终结果。
相关问题
c 语言计算char*占用字节数完整代码示例
下面是一个完整的示例代码,可以计算 char* 类型变量占用的字节数:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char* str = "Hello, world!";
int length = sizeof(str);
printf("'%s' 占用的字节数为: %d\n", str, length);
return 0;
}
```
该代码中,我们定义了一个 char* 类型的变量 str,其值为 "Hello, world!"。然后使用 sizeof() 函数来计算该变量占用的字节数,将结果保存在 length 变量中,并输出结果。注意,sizeof() 函数返回的是该变量的字节数,而不是字符串长度。
当输入字符串后,可以原样输出字符串,统计字符串占用几个字节;并统计字符串中大写字母字符多少个?小写字母字符多少个?数字字符多少个?空格多少个,其他字符多少个?
要实现输入字符串后输出原样字符串并统计各个字符的数量,你可以使用 C 语言的字符处理函数和循环来实现。以下是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
void count_characters(char* str) {
int uppercase_count = 0;
int lowercase_count = 0;
int digit_count = 0;
int space_count = 0;
int other_count = 0;
// 统计字符数量
for (int i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) {
uppercase_count++;
} else if (islower(str[i])) {
lowercase_count++;
} else if (isdigit(str[i])) {
digit_count++;
} else if (isspace(str[i])) {
space_count++;
} else {
other_count++;
}
}
printf("原样输出字符串: %s\n", str);
printf("字符串占用 %d 个字节\n", sizeof(str));
printf("大写字母字符数量: %d\n", uppercase_count);
printf("小写字母字符数量: %d\n", lowercase_count);
printf("数字字符数量: %d\n", digit_count);
printf("空格数量: %d\n", space_count);
printf("其他字符数量: %d\n", other_count);
}
int main() {
char str[100];
printf("请输入一个字符串: ");
scanf("%s", str);
count_characters(str);
return 0;
}
```
这段代码会接受用户输入的字符串,并统计其中大写字母、小写字母、数字、空格和其他字符的数量。然后,它会原样输出输入的字符串,并打印出字符串占用的字节数。你可以根据需要对代码进行修改和扩展。
阅读全文
相关推荐
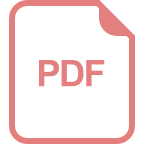
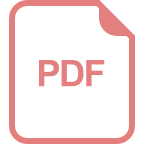
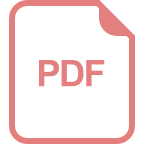
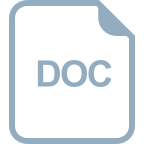



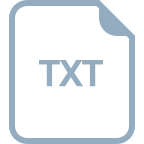
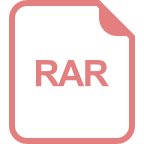
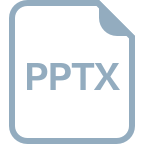
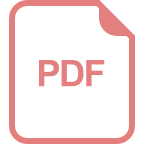
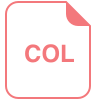




