用c语言:叉树采用二叉链表存储结构,试写算法求二叉树中一个非根结点的双亲结点(该非根结点的值由形参给出)。
时间: 2024-03-14 07:44:59 浏览: 27
下面是用C语言实现叉树二叉链表存储结构的算法,用于查找二叉树中一个非根结点的双亲结点。
```c
#include <stdio.h>
#include <stdlib.h>
// 叉树的二叉链表存储结构
typedef struct BiTNode {
int data;
struct BiTNode *lchild, *rchild;
} BiTNode, *BiTree;
// 查找二叉树中一个非根结点的双亲结点
BiTree find_parent_node(BiTree root, int x) {
// 如果根节点为空,则返回空
if (root == NULL) {
return NULL;
}
// 如果根节点的左孩子或右孩子的值为x,则返回根节点
if ((root->lchild && root->lchild->data == x) || (root->rchild && root->rchild->data == x)) {
return root;
}
// 在左子树中递归查找
BiTree parent = find_parent_node(root->lchild, x);
if (parent) {
return parent;
}
// 在右子树中递归查找
parent = find_parent_node(root->rchild, x);
if (parent) {
return parent;
}
return NULL; // 没有找到返回空
}
int main() {
// 构造一棵二叉树
BiTree root = (BiTree)malloc(sizeof(BiTNode));
root->data = 1;
root->lchild = (BiTree)malloc(sizeof(BiTNode));
root->lchild->data = 2;
root->lchild->lchild = NULL;
root->lchild->rchild = NULL;
root->rchild = (BiTree)malloc(sizeof(BiTNode));
root->rchild->data = 3;
root->rchild->lchild = (BiTree)malloc(sizeof(BiTNode));
root->rchild->lchild->data = 4;
root->rchild->lchild->lchild = NULL;
root->rchild->lchild->rchild = NULL;
root->rchild->rchild = (BiTree)malloc(sizeof(BiTNode));
root->rchild->rchild->data = 5;
root->rchild->rchild->lchild = NULL;
root->rchild->rchild->rchild = NULL;
// 查找二叉树中一个非根结点的双亲结点
BiTree parent = find_parent_node(root, 4);
if (parent) {
printf("The parent of node 4 is %d\n", parent->data);
} else {
printf("Node 4 is not in the tree or it is the root node.\n");
}
return 0;
}
```
在这个例子中,我们构造了一棵二叉树,并查找了结点4的双亲结点。输出结果为:
```
The parent of node 4 is 3
```
说明结点4的双亲结点是结点3。
相关推荐
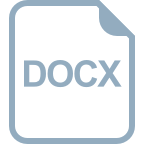














