使用C#语言写一段OPC UA客户端通讯读写数据
时间: 2024-04-08 15:33:15 浏览: 17
当使用C#语言编写OPC UA客户端通讯时,你可以使用OPC Foundation提供的UA-.NETStandard库来实现。下面是一个简单的示例代码,展示了如何使用C#语言读写OPC UA数据:
```csharp
using Opc.Ua;
using Opc.Ua.Client;
public class OpcUaClient
{
private Session session;
public void Connect(string endpointUrl)
{
// 创建一个UA应用程序实例
ApplicationInstance application = new ApplicationInstance();
application.ApplicationName = "OpcUaClient";
// 创建一个会话
session = Session.Create(
application.ApplicationConfiguration,
new ConfiguredEndpoint(null, new EndpointDescription(endpointUrl)),
false,
"",
60000,
new UserIdentity(new AnonymousIdentityToken()),
null).Result;
// 连接会话
session?.Open();
}
public void ReadNode(string nodeId)
{
// 读取节点的值
NodeId nodeToRead = new NodeId(nodeId);
DataValue value = session.ReadValue(nodeToRead);
Console.WriteLine("Value: " + value.Value);
}
public void WriteNode(string nodeId, object value)
{
// 写入节点的值
NodeId nodeToWrite = new NodeId(nodeId);
session.WriteValue(nodeToWrite, value);
}
public void Disconnect()
{
// 断开连接并释放资源
session?.Close();
session?.Dispose();
}
}
// 使用示例
public class Program
{
public static void Main(string[] args)
{
string endpointUrl = "opc.tcp://localhost:4840"; // OPC UA服务器的URL
string nodeId = "ns=2;s=Sensor.Value"; // 要读写的节点ID
OpcUaClient client = new OpcUaClient();
client.Connect(endpointUrl);
// 读取节点的值
client.ReadNode(nodeId);
// 写入节点的值
client.WriteNode(nodeId, 123);
client.Disconnect();
}
}
```
请注意,以上代码仅作为示例,实际使用时可能需要根据具体情况进行修改和扩展。你需要将代码中的`endpointUrl`替换为你要连接的OPC UA服务器的URL,`nodeId`替换为你要读写的节点ID。此外,你还需要在项目中引用`Opc.Ua.Core`和`Opc.Ua.Client`命名空间。
希望这段代码能帮助你开始使用C#语言进行OPC UA客户端通讯。如有任何问题,请随时提问。
相关推荐
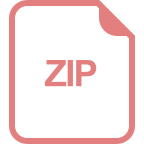
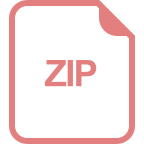














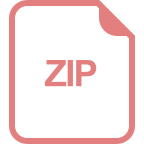