springboot基于shiro配置文件
时间: 2023-09-09 22:12:14 浏览: 61
在Spring Boot项目中使用Shiro进行安全控制,需要进行以下几个步骤。
1. 引入Shiro和Spring Boot相关依赖
在`pom.xml`文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring</artifactId>
<version>1.6.0</version>
</dependency>
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-web</artifactId>
<version>1.6.0</version>
</dependency>
```
2. 配置Shiro的安全管理器
在Spring Boot的配置文件中,添加Shiro的相关配置:
```yaml
# Shiro配置
shiro:
# 配置安全管理器
securityManager:
# 配置Realm
realms:
- name: myRealm
# 配置自定义的Realm实现类
customRealm: com.example.demo.shiro.MyRealm
# 配置Session管理器
sessionManager:
# Session过期时间,单位:毫秒
globalSessionTimeout: 1800000
# 配置Cookie
cookie:
# Cookie名称
name: SHIROSESSIONID
# Cookie过期时间,单位:秒
maxAge: 1800
# Cookie路径
path: /
# 配置Shiro Filter
filters:
# 配置自定义的Filter实现类
myFilter: com.example.demo.shiro.MyFilter
# 配置Filter Chain
filterChainDefinitions:
# 配置访问控制规则
/login: anon
/logout: logout
/**: myFilter
```
其中,配置了安全管理器`securityManager`,包括了自定义的Realm实现类`MyRealm`、Session管理器和Cookie。
配置了Shiro Filter`myFilter`,以及Filter Chain,其中`/login`可以匿名访问,`/logout`需要进行登出操作,其他URL需要经过自定义的Filter`MyFilter`进行访问控制。
3. 实现自定义Realm
在`MyRealm`类中,需要实现Shiro的`Realm`接口,重写`doGetAuthenticationInfo`和`doGetAuthorizationInfo`方法,完成身份认证和授权。
```java
public class MyRealm extends AuthorizingRealm {
/**
* 身份认证
*/
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken authenticationToken) throws AuthenticationException {
// 获取用户名和密码
String username = (String) authenticationToken.getPrincipal();
String password = new String((char[]) authenticationToken.getCredentials());
// 根据用户名查询用户信息
User user = userService.findByUsername(username);
// 判断用户是否存在
if (user == null) {
throw new UnknownAccountException("用户不存在");
}
// 判断密码是否正确
if (!password.equals(user.getPassword())) {
throw new IncorrectCredentialsException("密码错误");
}
// 将用户信息封装到AuthenticationInfo中
SimpleAuthenticationInfo authenticationInfo = new SimpleAuthenticationInfo(user, password, getName());
return authenticationInfo;
}
/**
* 授权
*/
@Override
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principalCollection) {
// 获取用户信息
User user = (User) principalCollection.getPrimaryPrincipal();
// 查询用户角色和权限信息
List<Role> roles = roleService.findByUserId(user.getId());
List<Permission> permissions = permissionService.findByUserId(user.getId());
// 封装角色和权限信息到AuthorizationInfo中
SimpleAuthorizationInfo authorizationInfo = new SimpleAuthorizationInfo();
for (Role role : roles) {
authorizationInfo.addRole(role.getName());
}
for (Permission permission : permissions) {
authorizationInfo.addStringPermission(permission.getName());
}
return authorizationInfo;
}
}
```
4. 实现自定义Filter
在`MyFilter`类中,需要继承Shiro的`AccessControlFilter`类,重写`isAccessAllowed`和`onAccessDenied`方法,完成访问控制逻辑。
```java
public class MyFilter extends AccessControlFilter {
@Override
protected boolean isAccessAllowed(ServletRequest servletRequest, ServletResponse servletResponse, Object o) throws Exception {
// 获取Shiro的Subject对象
Subject subject = getSubject(servletRequest, servletResponse);
// 判断是否已经登录
if (subject.isAuthenticated()) {
return true;
}
// 判断是否是登录请求
HttpServletRequest request = (HttpServletRequest) servletRequest;
if ("/login".equals(request.getServletPath())) {
return true;
}
// 其他情况都要进行跳转到登录页面
redirectToLogin(servletRequest, servletResponse);
return false;
}
@Override
protected boolean onAccessDenied(ServletRequest servletRequest, ServletResponse servletResponse) throws Exception {
// 如果是Ajax请求,则返回JSON格式的数据
HttpServletRequest request = (HttpServletRequest) servletRequest;
HttpServletResponse response = (HttpServletResponse) servletResponse;
if (request.getHeader("X-Requested-With") != null && request.getHeader("X-Requested-With").equalsIgnoreCase("XMLHttpRequest")) {
response.setContentType("application/json;charset=UTF-8");
response.getWriter().write(JSON.toJSONString(Result.fail("未登录或登录超时")));
} else {
// 否则进行跳转到登录页面
redirectToLogin(servletRequest, servletResponse);
}
return false;
}
}
```
5. 配置Shiro的注解支持
在Spring Boot的配置类中,添加`@EnableAspectJAutoProxy(proxyTargetClass = true)`注解和`@EnableAuthorization`注解,启用Shiro的注解支持和AOP功能。
```java
@Configuration
@EnableAspectJAutoProxy(proxyTargetClass = true)
@EnableAuthorization
public class ShiroConfig {
// ...
}
```
至此,基于Shiro的安全控制已经配置完成。在需要进行访问控制的Controller方法上,添加相应的注解即可完成授权操作,例如`@RequiresPermissions("user:view")`表示需要拥有`user:view`权限才能访问该方法。
相关推荐
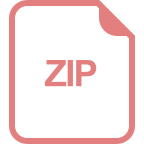
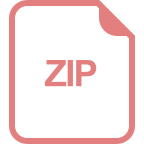
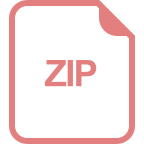














