pymongo筛选条件高级语句
时间: 2023-06-28 08:13:11 浏览: 134
在使用pymongo进行查询时,可以使用比较运算符、逻辑运算符、正则表达式等高级语句来筛选数据。
比较运算符:
1. $eq:等于
2. $ne:不等于
3. $gt:大于
4. $gte:大于等于
5. $lt:小于
6. $lte:小于等于
例如:
```python
from pymongo import MongoClient
client = MongoClient()
db = client.test_db
collection = db.test_collection
# 查询age等于18的记录
result = collection.find({"age": {"$eq": 18}})
# 查询age大于等于18的记录
result = collection.find({"age": {"$gte": 18}})
```
逻辑运算符:
1. $and:与
2. $or:或
3. $not:非
例如:
```python
from pymongo import MongoClient
client = MongoClient()
db = client.test_db
collection = db.test_collection
# 查询age等于18并且gender等于"male"的记录
result = collection.find({"$and": [{"age": 18}, {"gender": "male"}]})
# 查询age小于18或gender等于"female"的记录
result = collection.find({"$or": [{"age": {"$lt": 18}}, {"gender": "female"}]})
```
正则表达式:
可以使用正则表达式来匹配文本字段。
例如:
```python
from pymongo import MongoClient
client = MongoClient()
db = client.test_db
collection = db.test_collection
# 查询name以"Tom"开头的记录
result = collection.find({"name": {"$regex": "^Tom"}})
```
其他高级语句还包括:$in、$nin、$exists、$type等,可以根据具体需求选择使用。
阅读全文
相关推荐
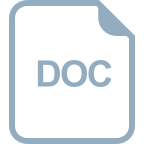
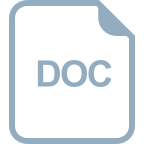
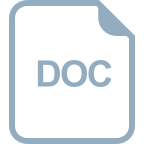
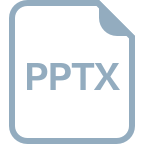
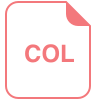
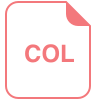
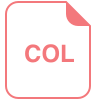
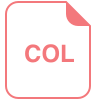
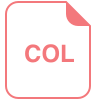
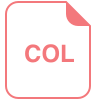
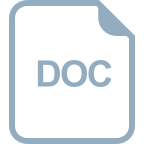
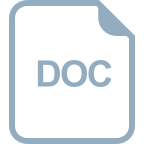
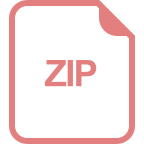
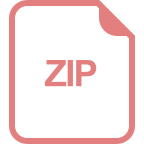
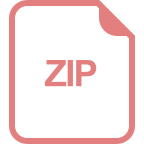
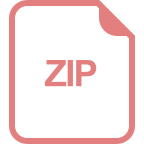
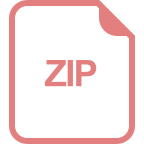
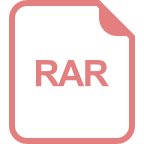