统计字符串中数字的个数(可使用正则进行验证)
时间: 2024-05-08 16:17:14 浏览: 8
可以使用正则表达式来匹配字符串中的数字,然后再统计数量。下面是一个Python的实现,其中使用了re模块的findall函数来匹配数字,然后使用len函数统计数量:
```python
import re
def count_numbers(s):
pattern = r'\d'
numbers = re.findall(pattern, s)
return len(numbers)
```
示例:
```python
s = 'abc123def456'
count = count_numbers(s)
print(count) # 输出:6
```
另外,如果只是想验证字符串中是否只包含数字,可以使用正则表达式的match函数:
```python
import re
def is_all_numbers(s):
pattern = r'^\d+$'
return bool(re.match(pattern, s))
```
示例:
```python
s1 = '12345'
s2 = '12a45'
print(is_all_numbers(s1)) # 输出:True
print(is_all_numbers(s2)) # 输出:False
```
相关问题
统计字符串中数字字符的个数
好的,下面是使用 C/C++ 实现统计字符串中数字字符的个数的代码:
使用指针:
```c
#include <stdio.h>
int count_digits(char* str){
int count = 0;
while(*str != '\0'){
if(*str >= '0' && *str <= '9'){
count++;
}
str++;
}
return count;
}
int main(){
char str[100];
printf("请输入字符串:\n");
scanf("%s", str);
printf("字符串中数字字符的个数为:%d\n", count_digits(str));
return 0;
}
```
在 `count_digits` 函数中,使用指针遍历字符串中的每一个字符,如果该字符是数字字符,则计数器加一。最后返回计数器的值。
使用引用:
```c++
#include <iostream>
#include <string>
using namespace std;
int count_digits(string& str){
int count = 0;
for(char c : str){
if(c >= '0' && c <= '9'){
count++;
}
}
return count;
}
int main(){
string str;
cout << "请输入字符串:" << endl;
cin >> str;
cout << "字符串中数字字符的个数为:" << count_digits(str) << endl;
return 0;
}
```
在 `count_digits` 函数中,使用 `for` 循环遍历字符串中的每一个字符,如果该字符是数字字符,则计数器加一。最后返回计数器的值。
统计字符串中数字的个数
可以使用循环和isdigit()函数来统计字符串中数字的个数,具体代码如下:
```python
s = input("请输入字符串:")
count = 0
for c in s:
if c.isdigit():
count += 1
print("字符串中数字的个数为:", count)
```
其中,isdigit()函数用于判断一个字符是否为数字。在循环中,依次遍历字符串中的每一个字符,如果是数字,则计数器加1。最后输出计数器的值即可。
相关推荐
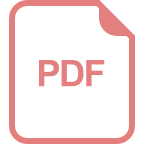
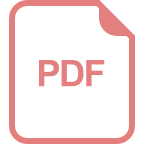












