分别使用pickle和struct 写入和读取二进制文件。
时间: 2023-08-09 18:06:23 浏览: 93
`pickle`和`struct`是Python标准库中用于序列化和反序列化数据的模块。`pickle`模块可以将Python对象转换为字节流,以便于存储或传输,而`struct`模块可以将Python数据按指定格式打包成字节流,或将字节流按指定格式解包成Python数据。
下面分别使用`pickle`和`struct`模块,实现写入和读取二进制文件的示例:
使用`pickle`模块写入二进制文件:
```python
import pickle
def write_with_pickle(data, file_path):
with open(file_path, 'wb') as f:
pickle.dump(data, f)
# 示例用法
data = [1, 2, 3, 4, 5]
file_path = "data.pkl"
write_with_pickle(data, file_path)
```
在上面的代码中,`with open()`语句用于打开二进制文件,`mode='wb'`参数指定打开模式为写二进制(write binary)模式。函数`write_with_pickle()`接收一个数据对象和一个文件路径作为参数,将数据对象使用`pickle.dump()`函数序列化为二进制流,然后写入文件中。
使用`pickle`模块读取二进制文件:
```python
import pickle
def read_with_pickle(file_path):
with open(file_path, 'rb') as f:
data = pickle.load(f)
return data
# 示例用法
file_path = "data.pkl"
data = read_with_pickle(file_path)
print(data)
```
在上面的代码中,`with open()`语句用于打开二进制文件,`mode='rb'`参数指定打开模式为读二进制(read binary)模式。函数`read_with_pickle()`接收一个文件路径作为参数,使用`pickle.load()`函数从文件中反序列化出数据对象,然后返回该对象。
使用`struct`模块写入二进制文件:
```python
import struct
def write_with_struct(data, file_path):
with open(file_path, 'wb') as f:
# 将数据按指定格式打包成二进制流
packed_data = struct.pack('i' * len(data), *data)
f.write(packed_data)
# 示例用法
data = [1, 2, 3, 4, 5]
file_path = "data.bin"
write_with_struct(data, file_path)
```
在上面的代码中,`with open()`语句用于打开二进制文件,`mode='wb'`参数指定打开模式为写二进制(write binary)模式。函数`write_with_struct()`接收一个数据对象和一个文件路径作为参数,将数据对象按指定格式(这里使用'i'表示整数)打包成二进制流,然后写入文件中。
使用`struct`模块读取二进制文件:
```python
import struct
def read_with_struct(file_path):
with open(file_path, 'rb') as f:
# 读取整个文件内容,并按指定格式解包成数据
file_content = f.read()
data = struct.unpack('i' * (len(file_content) // 4), file_content)
return data
# 示例用法
file_path = "data.bin"
data = read_with_struct(file_path)
print(data)
```
在上面的代码中,`with open()`语句用于打开二进制文件,`mode='rb'`参数指定打开模式为读二进制(read binary)模式。函数`read_with_struct()`接收一个文件路径作为参数,使用`f.read()`函数读取整个文件内容,并按指定格式(这里使用'i'表示整数)解包成数据对象,然后返回该对象。
阅读全文
相关推荐
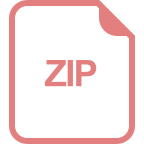
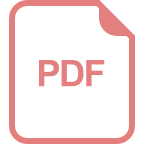





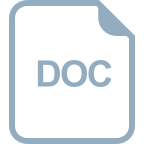
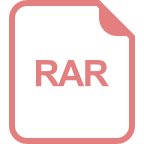
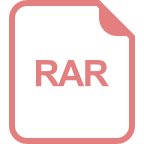
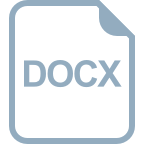
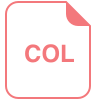
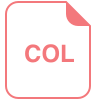
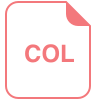
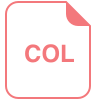
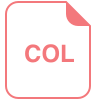
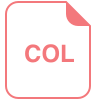
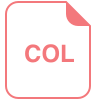