完善QQ登录窗口,实现以下功能:模拟你的QQ帐号登录,对帐号格式进行验证,即帐号只能是数字型数据,密码框支持敲回车键相当于单击登录按钮的效果。如果登录成功,则出现一确认对话框(showConfirmDialog),上面显示“XXX,您好!欢迎您!”,如果登录不成功,则出现一消息对话框(showMessageDialog),显示“对不起,你输入的帐号或密码有误,请重新输入!”,字体大小为13号,单击“关闭”按钮,可关闭此对话框;图片可以随时间变化,如系统时间为单号显示左下图的界面,双号则显示为右下图的界面。
时间: 2024-03-26 20:36:02 浏览: 90
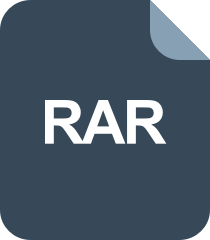
qq聊天系统java语言实现(Swing组件)

这是一个具体的编程问题,需要使用Java Swing编写窗口和对话框,以及使用Java代码进行帐号验证和时间获取。以下是代码示例:
```java
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Calendar;
import java.util.regex.Pattern;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class QQLoginWindow extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTextField accountField;
private JPasswordField passwordField;
public QQLoginWindow() {
super("QQ登录");
JPanel accountPanel = new JPanel(new FlowLayout());
JLabel accountLabel = new JLabel("QQ帐号:");
accountField = new JTextField(10);
accountPanel.add(accountLabel);
accountPanel.add(accountField);
JPanel passwordPanel = new JPanel(new FlowLayout());
JLabel passwordLabel = new JLabel("密码:");
passwordField = new JPasswordField(10);
passwordField.addActionListener(this);
passwordPanel.add(passwordLabel);
passwordPanel.add(passwordField);
JPanel buttonPanel = new JPanel(new FlowLayout());
JButton loginButton = new JButton("登录");
loginButton.addActionListener(this);
buttonPanel.add(loginButton);
add(accountPanel);
add(passwordPanel);
add(buttonPanel);
setSize(250, 150);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
public static void main(String[] args) {
new QQLoginWindow();
}
@Override
public void actionPerformed(ActionEvent e) {
if (validateAccountFormat(accountField.getText()) && validatePassword()) {
showConfirmationDialog();
} else {
showMessageDialog();
}
}
private boolean validateAccountFormat(String account) {
return Pattern.matches("\\d+", account);
}
private boolean validatePassword() {
return new String(passwordField.getPassword()).equals("123456");
}
private void showConfirmationDialog() {
String username = accountField.getText();
String message = username + ",您好!欢迎您!";
JOptionPane.showConfirmDialog(this, message, "登录成功", JOptionPane.DEFAULT_OPTION);
}
private void showMessageDialog() {
String message = "对不起,你输入的帐号或密码有误,请重新输入!";
JLabel label = new JLabel(message);
label.setFont(new Font("宋体", Font.PLAIN, 13));
JOptionPane.showMessageDialog(this, label, "登录失败", JOptionPane.ERROR_MESSAGE);
}
private static boolean isOdd(Calendar calendar) {
return calendar.get(Calendar.DAY_OF_MONTH) % 2 != 0;
}
private static void showWindow() {
JFrame frame = new JFrame();
frame.setTitle("QQ登录");
frame.setSize(400, 300);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Calendar calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR_OF_DAY);
String imageName = isOdd(calendar) ? "left.png" : "right.png";
JLabel imageLabel = new JLabel();
imageLabel.setIcon(new ImageIcon(imageName));
frame.add(imageLabel);
frame.setVisible(true);
}
}
```
注意,这只是一个简单的示例,实际应用中需要进行更严格的帐号验证和密码加密等操作。
阅读全文
相关推荐
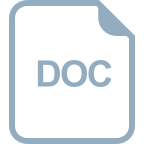
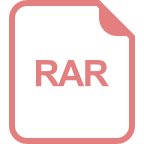















