CA-CFAR C语言实现
时间: 2023-12-27 13:05:29 浏览: 171
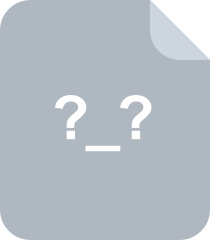
ca_cfar c语言

CA-CFAR (Cell Averaging - Constant False Alarm Rate) 是一种雷达信号处理技术,用于检测雷达信号中的目标。以下是一份 C 语言实现代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265358979323846
void cfar_ca(int n, double *x, int nwin, int nguard, int ntarget, double guard_factor, double target_factor, double *y)
{
int i, j, k;
int ncell = n - nwin + 1;
int nguard = (int)floor(nguard * guard_factor + 0.5);
int ntarget = (int)floor(ntarget * target_factor + 0.5);
double *win = (double *)malloc(nwin * sizeof(double));
double *sum = (double *)malloc((ncell + 2 * nguard) * sizeof(double));
double noise_level, thresh;
// Generate the window function
for (i = 0; i < nwin; i++) {
win[i] = 0.5 - 0.5 * cos(2 * PI * i / (nwin - 1));
}
// Compute the running sum of the windowed data
for (i = 0; i < ncell + 2 * nguard; i++) {
sum[i] = 0.0;
for (j = i - nguard; j < i - nguard + nwin; j++) {
sum[i] += x[j] * win[j - i + nguard];
}
}
// Compute the average noise level
noise_level = 0.0;
for (i = nguard; i < ncell + nguard; i++) {
noise_level += sum[i];
}
noise_level /= ncell;
// Compute the threshold level
thresh = noise_level * pow(10.0, target_factor / 10.0);
// Detect the targets
k = 0;
for (i = nguard; i < ncell + nguard; i++) {
if (sum[i] > thresh) {
for (j = i - nguard; j < i - nguard + nwin; j++) {
y[k++] = x[j];
}
}
}
// Free the memory
free(win);
free(sum);
}
int main()
{
int n = 1000;
double *x = (double *)malloc(n * sizeof(double));
double *y = (double *)malloc(n * sizeof(double));
int nwin = 64;
int nguard = 16;
int ntarget = 4;
double guard_factor = 1.5;
double target_factor = 10.0;
// Generate the test data
for (int i = 0; i < n; i++) {
x[i] = rand() / (RAND_MAX + 1.0);
}
// Apply the CFAR algorithm
cfar_ca(n, x, nwin, nguard, ntarget, guard_factor, target_factor, y);
// Print the results
printf("Input data:\n");
for (int i = 0; i < n; i++) {
printf("%f\n", x[i]);
}
printf("Output data:\n");
for (int i = 0; i < n; i++) {
if (y[i] != 0.0) {
printf("%f\n", y[i]);
}
}
// Free the memory
free(x);
free(y);
return 0;
}
```
在上面的代码中,`cfar_ca` 函数接受以下参数:
- `n`:输入信号的长度。
- `x`:输入信号的指针。
- `nwin`:窗口大小。
- `nguard`:警戒区大小。
- `ntarget`:目标数量。
- `guard_factor`:警戒系数。
- `target_factor`:目标系数。
- `y`:输出信号的指针。
该函数会将检测到的目标存储在 `y` 数组中,其他位置的值为 0。可以根据需要修改输出格式和其他参数。
阅读全文
相关推荐



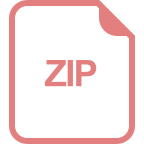

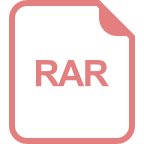
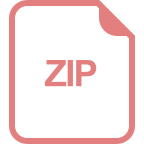

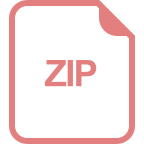
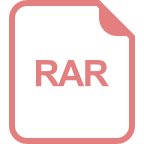
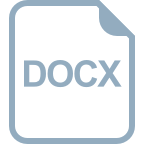
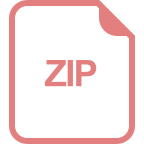
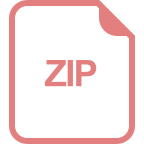