C#读取指定长度字符串
时间: 2023-09-17 17:11:04 浏览: 74
您可以使用 C# 中的字符串的 `Substring()` 方法来读取指定长度的字符串。该方法需要两个参数:起始位置和要读取的字符数。例如,如果您想要从一个字符串中读取前5个字符,可以使用以下代码:
```csharp
string str = "Hello World";
string subStr = str.Substring(0, 5); // subStr = "Hello"
```
在这个例子中,`Substring()` 方法的第一个参数是起始位置,即0,表示从字符串的第一个字符开始读取。第二个参数是要读取的字符数,即5,表示读取从起始位置开始的5个字符。
相关问题
c#怎么读取txt指定字符串前后制定长度的字符
你可以使用C#中的`StreamReader`类来读取文本文件,并使用字符串的`Substring`方法来截取指定长度的字符。下面是一个示例代码:
```csharp
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = "path/to/your/file.txt";
string searchStr = "target";
int lengthBefore = 5; // 指定字符串前的长度
int lengthAfter = 5; // 指定字符串后的长度
using (StreamReader sr = new StreamReader(filePath))
{
string line;
while ((line = sr.ReadLine()) != null)
{
int index = line.IndexOf(searchStr);
if (index != -1)
{
if (index >= lengthBefore && index + searchStr.Length + lengthAfter <= line.Length)
{
string result = line.Substring(index - lengthBefore, lengthBefore + searchStr.Length + lengthAfter);
Console.WriteLine(result);
}
}
}
}
}
}
```
在上面的示例中,你需要将`filePath`变量替换为你的文本文件的路径。`searchStr`是你要查找的目标字符串。`lengthBefore`和`lengthAfter`是指定的前后长度。当找到目标字符串时,通过使用`Substring`方法和位置索引,你可以截取出指定长度的字符并进行处理。
c#读取1200plc的字符串
要读取1200系列PLC的字符串,可以使用S7.Net库来进行编程。以下是一个简单的C#示例代码,可以读取PLC中DB1的字符串数据:
```
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using S7.Net;
namespace ReadStringFromPLC
{
class Program
{
static void Main(string[] args)
{
// Create a new instance of the S7.Net library's Plc class
var plc = new Plc(CpuType.S71200, "192.168.0.1", 0, 1);
// Open the connection to the PLC
plc.Open();
// Read the string from DB1, starting at byte 0 and with a length of 20 bytes
string myString = plc.Read("DB1.DBX0.0", 20).ToString();
// Close the connection to the PLC
plc.Close();
// Output the string to the console
Console.WriteLine(myString);
}
}
}
```
在上面的代码中,我们首先实例化了一个Plc对象,指定了PLC的型号、IP地址和机架号槽号。然后,我们打开了PLC连接,并读取了DB1中的字符串数据,起始于字节0,长度为20个字节。最后,我们关闭了PLC连接,并输出了读取到的字符串数据到控制台。
相关推荐
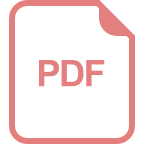
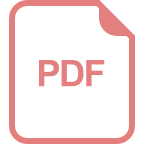
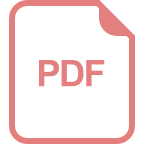












