写一个基于pytorch的LSTM模型代码 输入特征4 序列长度20 批次128
时间: 2023-03-06 16:11:33 浏览: 120
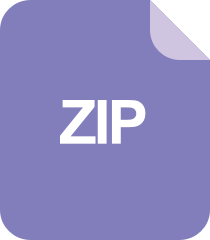
基于pytorch搭建多特征LSTM时间序列预测.zip

好的,以下是一个基于PyTorch的LSTM模型代码,输入特征为4,序列长度为20,批次为128。
```python
import torch
import torch.nn as nn
class LSTM(nn.Module):
def __init__(self, input_size=4, hidden_size=64, num_layers=1):
super(LSTM, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, 1)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
# 使用示例
model = LSTM(input_size=4, hidden_size=64, num_layers=1)
x = torch.randn(128, 20, 4) # 输入数据为 128 个样本,每个样本有 20 个时间步长,每个时间步长有 4 个特征
output = model(x)
print(output.shape) # 输出为 (128, 1),表示 128 个样本的预测结果
```
以上代码定义了一个LSTM模型类 `LSTM`,其中 `input_size` 为输入特征数,`hidden_size` 为 LSTM 隐藏层的大小,`num_layers` 为 LSTM 的层数。在 `forward` 函数中,首先将输入数据通过 LSTM 层进行处理,然后将最后一个时间步的输出通过全连接层 `fc` 得到预测结果。
在使用示例中,我们创建了一个大小为 (128, 20, 4) 的输入张量 `x`,其中第一维表示批次大小为 128,第二维表示序列长度为 20,第三维表示输入特征数为 4。将 `x` 作为输入传入模型 `model` 中,得到输出张量 `output`,其大小为 (128, 1),表示对于每个样本,预测结果为一个标量。
阅读全文
相关推荐
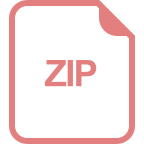





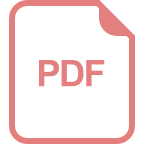
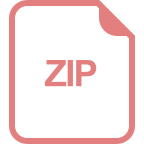
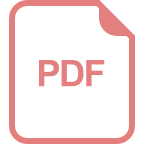
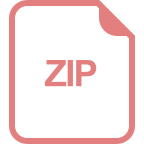
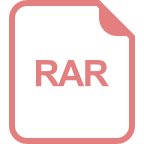
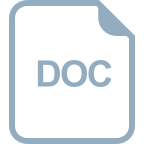
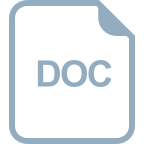


