#include<stdio.h> #include<string.h> #include<stdlib.h> int main(void) { FILE* fp1, * fp2; errno_t err1, err2; char ch, ch1, ch2; if ((err1 = fopen_s(&fp1, "test.txt", "r")) != 0) { printf("Open 1 fail!\n"); exit(0); } if ((err2 = fopen_s(&fp2, "test2.txt", "w")) != 0) { printf("Open 1 fail!\n"); exit(0); } while (!feof(fp1)) { ch = fgetc(fp1); if (ch == '/') { if ((ch1 = fgetc(fp1) == '*')){ while (fgetc(fp1) != '*' && (ch1 = fgetc(fp1)) != '/'); } else if (ch1 == '/') { while ((ch2 = fgetc(fp1)) != '\n'); } } else if(ch!=EOF) fputc(ch,fp2); } if (fclose(fp1)) { printf("Close Fail!\n"); exit(0); } if (fclose(fp2)) { printf("Close Fail!\n"); exit(0); } return 0; }
时间: 2024-02-01 13:03:47 浏览: 27
以下是将给定的代码翻译成C++的结果:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
FILE* fp1, * fp2;
errno_t err1, err2;
char ch, ch1, ch2;
if ((err1 = fopen_s(&fp1, "test.txt", "r")) != 0) {
cout << "Open 1 fail!" << endl;
exit(0);
}
if ((err2 = fopen_s(&fp2, "test2.txt", "w")) != 0) {
cout << "Open 1 fail!" << endl;
exit(0);
}
while (!feof(fp1)) {
ch = fgetc(fp1);
if (ch == '/') {
if ((ch1 = fgetc(fp1) == '*')) {
while (fgetc(fp1) != '*' && (ch1 = fgetc(fp1)) != '/');
}
else if (ch1 == '/') {
while ((ch2 = fgetc(fp1)) != '\n');
}
}
else if (ch != EOF) {
fputc(ch, fp2);
}
}
if (fclose(fp1)) {
cout << "Close Fail!" << endl;
exit(0);
}
if (fclose(fp2)) {
cout << "Close Fail!" << endl;
exit(0);
}
return 0;
}
```
需要注意的是,这个代码使用了 C 语言的库函数和头文件。在 C++ 中,我们通常使用 `<iostream>` 和 `<fstream>` 来进行文件输入输出操作。因此,我们需要对代码做出一些修改。
相关推荐
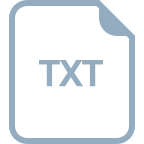
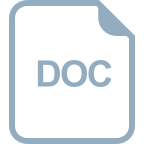
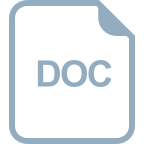














