int32_t main() { int t; cin >> t; while (t--) { int n, k; cin >> n >> k; k = min(k, 30); cout << min(n, (1 << k) - 1) + 1 << "\n"; } return 0; }用c语言来写
时间: 2024-03-14 21:44:59 浏览: 141
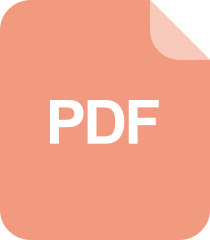
c++编程练习 030:你真的搞清楚为啥 while(cin >> n) 能成立了吗?

以下是使用 C 语言来实现这段代码的示例:
```c
#include <stdio.h>
#include <stdint.h>
int32_t main() {
int t;
scanf("%d", &t);
while (t--) {
int n, k;
scanf("%d %d", &n, &k);
k = k < 30 ? k : 30;
printf("%d\n", (n < ((1 << k) - 1)) ? n + 1 : ((1 << k) - 1) + 1);
}
return 0;
}
```
这段代码与上述 C++ 代码的功能相同。首先,它读入一个整数 t,表示测试用例的数量。然后,使用 while 循环遍历 t 次,每次读入两个整数 n 和 k,表示问题的输入数据。接着,使用三目运算符计算满足条件的整数值,并输出到控制台。需要注意的是,在 C 语言中,需要使用 scanf 和 printf 函数来进行输入输出。
阅读全文
相关推荐
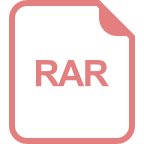
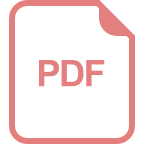
















