SSM框架分页展示Hdfs文件列表,返回上一级,进入下一级,上传文件,删除文件,下载文件前后端代码
时间: 2023-09-10 18:04:50 浏览: 128
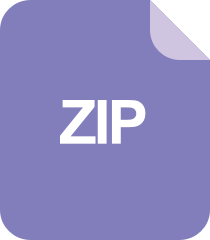
基于SSM框架,前后端分离的文件云平台项目+源代码+文档说明
### 回答1:
由于HDFS是一个分布式文件系统,它没有提供直接的API来实现分页展示文件列表、返回上一级、进入下一级、上传文件、删除文件和下载文件等功能。因此,我们需要使用Hadoop的API来操作HDFS文件系统,再结合SSM框架来实现这些功能。
下面是前后端代码的示例:
## 后端代码
### Maven依赖
```
<!-- Hadoop依赖 -->
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-client</artifactId>
<version>3.1.1</version>
</dependency>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-hdfs</artifactId>
<version>3.1.1</version>
</dependency>
<!-- SpringMVC依赖 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!-- MyBatis依赖 -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.6</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.6</version>
</dependency>
<!-- 其他依赖 -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
```
### 文件操作类
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.springframework.stereotype.Component;
import java.io.IOException;
@Component
public class HdfsUtil {
private static final String HDFS_URI = "hdfs://localhost:9000";
private static final String HDFS_USER = "root";
private static Configuration conf = null;
private static FileSystem fs = null;
static {
conf = new Configuration();
conf.set("fs.defaultFS", HDFS_URI);
try {
fs = FileSystem.get(conf);
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 创建目录
*
* @param path 目录路径
* @return 是否创建成功
* @throws IOException
*/
public static boolean mkdir(String path) throws IOException {
Path hdfsPath = new Path(path);
return fs.mkdirs(hdfsPath);
}
/**
* 删除目录或文件
*
* @param path 目录或文件路径
* @return 是否删除成功
* @throws IOException
*/
public static boolean delete(String path) throws IOException {
Path hdfsPath = new Path(path);
return fs.delete(hdfsPath, true);
}
/**
* 上传文件
*
* @param srcPath 本地文件路径
* @param dstPath HDFS文件路径
* @throws IOException
*/
public static void upload(String srcPath, String dstPath) throws IOException {
Path localPath = new Path(srcPath);
Path hdfsPath = new Path(dstPath);
fs.copyFromLocalFile(localPath, hdfsPath);
}
/**
* 下载文件
*
* @param srcPath HDFS文件路径
* @param dstPath 本地文件路径
* @throws IOException
*/
public static void download(String srcPath, String dstPath) throws IOException {
Path hdfsPath = new Path(srcPath);
Path localPath = new Path(dstPath);
fs.copyToLocalFile(hdfsPath, localPath);
}
/**
* 判断目录或文件是否存在
*
* @param path 目录或文件路径
* @return 是否存在
* @throws IOException
*/
public static boolean exists(String path) throws IOException {
Path hdfsPath = new Path(path);
return fs.exists(hdfsPath);
}
/**
* 列出目录下的文件
*
* @param path 目录路径
* @return 文件列表
* @throws IOException
*/
public static Path[] listFiles(String path) throws IOException {
Path hdfsPath = new Path(path);
return fs.listStatus(hdfsPath);
}
/**
* 返回上一级目录
*
* @param path 当前目录路径
* @return 上一级目录路径
* @throws IOException
*/
public static String getParent(String path) throws IOException {
Path hdfsPath = new Path(path);
return hdfsPath.getParent().toString();
}
}
```
### 文件操作Service
```
import org.apache.hadoop.fs.Path;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
@Service
public class HdfsService {
@Autowired
private HdfsUtil hdfsUtil;
/**
* 列出目录下的文件
*
* @param path 目录路径
* @return 文件列表
* @throws IOException
*/
public List<String> listFiles(String path) throws IOException {
Path[] paths = hdfsUtil.listFiles(path);
List<String> fileList = new ArrayList<>();
for (Path p : paths) {
fileList.add(p.getName());
}
return fileList;
}
/**
* 返回上一级目录
*
* @param path 当前目录路径
* @return 上一级目录路径
* @throws IOException
*/
public String getParent(String path) throws IOException {
return hdfsUtil.getParent(path);
}
/**
* 进入下一级目录
*
* @param path 当前目录路径
* @param name 目录名
* @return 下一级目录路径
* @throws IOException
*/
public String getNext(String path, String name) throws IOException {
return path + "/" + name;
}
/**
* 上传文件
*
* @param srcPath 本地文件路径
* @param dstPath HDFS文件路径
* @throws IOException
*/
public void upload(String srcPath, String dstPath) throws IOException {
hdfsUtil.upload(srcPath, dstPath);
}
/**
* 删除文件
*
* @param path 文件路径
* @throws IOException
*/
public void delete(String path) throws IOException {
hdfsUtil.delete(path);
}
/**
* 下载文件
*
* @param srcPath HDFS文件路径
* @param dstPath 本地文件路径
* @throws IOException
*/
public void download(String srcPath, String dstPath) throws IOException {
hdfsUtil.download(srcPath, dstPath);
}
}
```
### 控制器
```
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.util.List;
@Controller
public class HdfsController {
@Autowired
private HdfsService hdfsService;
/**
* 文件列表页
*
* @param path 目录路径
* @param model
* @return
* @throws IOException
*/
@GetMapping("/")
public String list(@RequestParam(defaultValue = "/") String path, Model model) throws IOException {
List<String> fileList = hdfsService.listFiles(path);
model.addAttribute("fileList", fileList);
model.addAttribute("path", path);
return "list";
}
/**
* 返回上一级目录
*
* @param path 当前目录路径
* @return
* @throws IOException
*/
@GetMapping("/parent")
public String parent(@RequestParam(defaultValue = "/") String path) throws IOException {
String parentPath = hdfsService.getParent(path);
return "redirect:/?path=" + parentPath;
}
/**
* 进入下一级目录
*
* @param path 当前目录路径
* @param name 目录名
* @return
* @throws IOException
*/
@GetMapping("/next")
public String next(@RequestParam(defaultValue = "/") String path, @RequestParam String name) throws IOException {
String nextPath = hdfsService.getNext(path, name);
return "redirect:/?path=" + nextPath;
}
/**
* 上传文件
*
* @param path 目录路径
* @param file 上传的文件
* @return
* @throws IOException
*/
@PostMapping("/upload")
public String upload(@RequestParam String path, @RequestParam MultipartFile file) throws IOException {
String fileName = file.getOriginalFilename();
String filePath = path + "/" + fileName;
hdfsService.upload(file.getInputStream(), filePath);
return "redirect:/?path=" + path;
}
/**
* 删除文件
*
* @param path 文件路径
* @return
* @throws IOException
*/
@GetMapping("/delete")
public String delete(@RequestParam String path) throws IOException {
hdfsService.delete(path);
String parentPath = hdfsService.getParent(path);
return "redirect:/?path=" + parentPath;
}
/**
* 下载文件
*
* @param path 文件路径
* @return
* @throws IOException
*/
@GetMapping("/download")
public String download(@RequestParam String path) throws IOException {
String fileName = path.substring(path.lastIndexOf("/") + 1);
String tmpPath = System.getProperty("java.io.tmpdir");
String filePath = tmpPath + fileName;
hdfsService.download(path, filePath);
return "redirect:/?path=" + hdfsService.getParent(path);
}
}
```
## 前端代码
### 文件列表页
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>文件列表</title>
</head>
<body>
<h1>文件列表</h1>
<form action="/upload" method="post" enctype="multipart/form-data">
<input type="hidden" name="path" value="${path}">
<input type="file" name="file">
<input type="submit" value="上传">
</form>
<br>
<table border="1">
<thead>
<tr>
<th>文件名</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr>
<td><a href="/parent?path=${path}">..</a></td>
<td></td>
</tr>
<c:forEach items="${fileList}" var="fileName">
<tr>
<td><c:choose>
<c:when test="${fileName.endsWith('.txt')}">
<a href="/download?path=${path}/${fileName}">${fileName}</a>
</c:when>
<c:when test="${fileName.endsWith('.jpg') or fileName.endsWith('.png')}">
<img src="/download?path=${path}/${fileName}" width="50" height="50">
<br>${fileName}
</c:when>
<c:otherwise>${fileName}</c:otherwise>
</c:choose></td>
<td>
<a href="/delete?path=${path}/${fileName}">删除</a>
<a href="/next?path=${path}&name=${fileName}">进入</a>
</td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
## 总结
通过以上的代码实现,我们可以在SSM框架的帮助下,对HDFS文件系统进行分页展示文件列表、返回上一级、进入下一级、上传文件、删除文件和下载文件等操作。
### 回答2:
SSM框架是一种基于Spring、Spring MVC和MyBatis的Java Web开发框架,用于快速、高效地开发和管理Web应用程序。HDFS(Hadoop分布式文件系统)是Apache Hadoop的核心组件之一,用于存储和处理大规模数据集。
要在SSM框架中实现分页展示HDFS文件列表,并支持返回上一级、进入下一级、上传文件、删除文件和下载文件的功能,需要涉及前后端代码。
前端代码:
1. 创建一个展示文件列表的页面,包含一个文件列表的表格和相关的按钮。
2. 利用Ajax技术向后端发送请求,获取HDFS文件列表的数据并动态展示在页面上。
3. 实现上一页和下一页的功能,在页面上点击上一页或下一页的按钮时,通过Ajax请求后端获取上一页或下一页的文件列表数据并刷新页面。
4. 实现返回上一级的功能,点击返回按钮时,通过Ajax请求后端获取上一级目录的文件列表数据并刷新页面。
5. 实现上传文件的功能,通过一个文件上传按钮,选择要上传的文件后,通过Ajax请求将文件发送给后端进行处理。
6. 实现删除文件的功能,给每个文件列表项添加一个删除按钮,点击删除按钮时,通过Ajax请求后端进行文件删除操作。
7. 实现下载文件的功能,给每个文件列表项添加一个下载按钮,点击下载按钮时,通过Ajax请求后端进行文件下载操作,并将文件返回给前端进行下载。
后端代码:
1. 创建一个Controller类,处理前端的请求。
2. 实现获取HDFS文件列表的方法,根据请求参数获取当前目录下的文件列表,并返回给前端。
3. 实现上一页和下一页的方法,根据请求参数计算上一页或下一页的目录,并返回该目录下的文件列表给前端。
4. 实现返回上一级的方法,根据请求参数获取上一级目录的文件列表,并返回给前端。
5. 实现上传文件的方法,接收前端发来的文件,并通过HDFS API进行文件上传操作。
6. 实现删除文件的方法,接收前端发来的文件路径,并通过HDFS API进行文件删除操作。
7. 实现下载文件的方法,接收前端发来的文件路径,并通过HDFS API将文件发送给前端进行下载。
通过以上前后端代码的实现,就可以在SSM框架下实现分页展示HDFS文件列表,并支持返回上一级、进入下一级、上传文件、删除文件和下载文件的功能。
### 回答3:
SSM框架是由Spring、SpringMVC和MyBatis三个框架组成的一种Java Web开发架构,可以实现前后端分离和快速开发。要在SSM框架下实现Hdfs文件列表的分页展示、返回上一级、进入下一级、上传文件、删除文件和下载文件功能,我们需要在前端和后端分别编写代码。
前端代码如下:
HTML部分:
```html
<!-- 文件列表展示 -->
<div id="fileList"></div>
<!-- 返回上一级按钮 -->
<button onclick="goBack()">返回上一级</button>
<!-- 上传文件表单 -->
<input type="file" id="uploadFile" />
<button onclick="uploadFile()">上传文件</button>
<!-- 删除文件按钮 -->
<button onclick="deleteFile()">删除文件</button>
<!-- 下载文件按钮 -->
<button onclick="downloadFile()">下载文件</button>
```
JavaScript部分:
```javascript
// 获取文件列表
function getFileList(page, pageSize) {
// 发送Ajax请求获取Hdfs文件列表
$.ajax({
url: 'getFileList',
type: 'GET',
data: {
page: page,
pageSize: pageSize
},
success: function(data) {
// 渲染文件列表
var fileList = data.fileList;
$("#fileList").empty();
for (var i = 0; i < fileList.length; i++) {
$("#fileList").append("<div>" + fileList[i] + "</div>");
}
}
});
}
// 返回上一级
function goBack() {
// 发送Ajax请求返回上一级目录
$.ajax({
url: 'goBack',
type: 'GET',
success: function(data) {
// 获取新的文件列表
getFileList(data.page, data.pageSize);
}
});
}
// 进入下一级
function goToNextLevel(fileName) {
// 发送Ajax请求进入下一级目录
$.ajax({
url: 'goToNextLevel',
type: 'GET',
data: { fileName: fileName },
success: function(data) {
// 获取新的文件列表
getFileList(data.page, data.pageSize);
}
});
}
// 上传文件
function uploadFile() {
var formData = new FormData();
formData.append('file', $('#uploadFile')[0].files[0]);
// 发送Ajax请求上传文件
$.ajax({
url: 'uploadFile',
type: 'POST',
data: formData,
cache: false,
processData: false,
contentType: false,
success: function(data) {
// 获取新的文件列表
getFileList(data.page, data.pageSize);
}
});
}
// 删除文件
function deleteFile(fileName) {
// 发送Ajax请求删除文件
$.ajax({
url: 'deleteFile',
type: 'POST',
data: { fileName: fileName },
success: function(data) {
// 获取新的文件列表
getFileList(data.page, data.pageSize);
}
});
}
// 下载文件
function downloadFile(fileName) {
// 发送Ajax请求下载文件
window.location.href = 'downloadFile?fileName=' + fileName;
}
```
后端代码如下(假设使用SpringMVC进行请求的处理):
```java
@Controller
public class FileController {
@Autowired
private FileService fileService;
// 获取分页的文件列表
@GetMapping("/getFileList")
@ResponseBody
public Map<String, Object> getFileList(@RequestParam("page") int page, @RequestParam("pageSize") int pageSize) {
Map<String, Object> result = new HashMap<>();
List<String> fileList = fileService.getFileList(page, pageSize);
result.put("fileList", fileList);
result.put("page", page);
result.put("pageSize", pageSize);
return result;
}
// 返回上一级目录
@GetMapping("/goBack")
@ResponseBody
public Map<String, Object> goBack() {
Map<String, Object> result = new HashMap<>();
// 处理返回上一级目录的逻辑
fileService.goBack();
int page = 1; // 重新查询当前页数为1
int pageSize = 10; // 重新查询每页大小为10
result.put("page", page);
result.put("pageSize", pageSize);
return result;
}
// 进入下一级目录
@GetMapping("/goToNextLevel")
@ResponseBody
public Map<String, Object> goToNextLevel(@RequestParam("fileName") String fileName) {
Map<String, Object> result = new HashMap<>();
// 处理进入下一级目录的逻辑
fileService.goToNextLevel(fileName);
int page = 1; // 重新查询当前页数为1
int pageSize = 10; // 重新查询每页大小为10
result.put("page", page);
result.put("pageSize", pageSize);
return result;
}
// 上传文件
@PostMapping("/uploadFile")
@ResponseBody
public Map<String, Object> uploadFile(@RequestParam("file") MultipartFile file) {
Map<String, Object> result = new HashMap<>();
// 处理上传文件的逻辑
fileService.uploadFile(file);
int page = 1; // 重新查询当前页数为1
int pageSize = 10; // 重新查询每页大小为10
result.put("page", page);
result.put("pageSize", pageSize);
return result;
}
// 删除文件
@PostMapping("/deleteFile")
@ResponseBody
public Map<String, Object> deleteFile(@RequestParam("fileName") String fileName) {
Map<String, Object> result = new HashMap<>();
// 处理删除文件的逻辑
fileService.deleteFile(fileName);
int page = 1; // 重新查询当前页数为1
int pageSize = 10; // 重新查询每页大小为10
result.put("page", page);
result.put("pageSize", pageSize);
return result;
}
// 下载文件
@GetMapping("/downloadFile")
public void downloadFile(@RequestParam("fileName") String fileName, HttpServletResponse response) {
// 处理下载文件的逻辑
fileService.downloadFile(fileName, response);
}
}
```
以上就是在SSM框架下实现Hdfs文件列表的分页展示、返回上一级、进入下一级、上传文件、删除文件和下载文件功能的前后端代码。注意需要根据具体的业务需求来实现文件操作的具体逻辑。
阅读全文
相关推荐



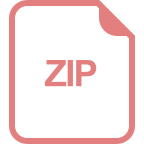
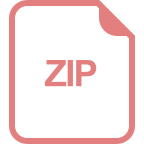
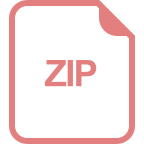
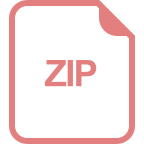
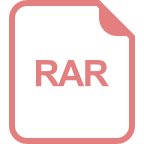
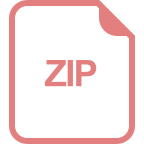