Java poi 实体类 填充excel模板
时间: 2023-08-28 21:04:47 浏览: 171
Java POI(Poor Obfuscation Implementation)是一个用于操作Microsoft Office文件格式的Java库。它支持从Excel文件中读取和写入数据,以及创建和修改Word和PowerPoint文档。
在Java POI中,我们可以使用实体类来填充Excel模板。实体类是一个Java类,其中包含了与Excel模板中每个单元格对应的属性。在填充过程中,我们可以通过对实体类属性进行赋值,将数据填充到Excel模板中。
以下是一个示例实体类的代码:
```
public class Student {
private String name;
private int age;
private String gender;
private double score;
// getters and setters
// ...
// constructor
public Student(String name, int age, String gender, double score) {
this.name = name;
this.age = age;
this.gender = gender;
this.score = score;
}
}
```
在Excel模板中,我们可以使用占位符来标记需要填充数据的单元格。例如,我们可以在单元格中输入“{{name}}”来表示该单元格需要填充学生姓名。
接下来,我们可以使用Java POI来加载Excel模板文件,并使用实体类来填充数据。以下是一个示例代码:
```
public class ExcelFiller {
public static void main(String[] args) throws IOException, InvalidFormatException {
// 加载Excel模板
Workbook workbook = WorkbookFactory.create(new File("template.xlsx"));
Sheet sheet = workbook.getSheetAt(0);
// 填充数据
Student student = new Student("Tom", 18, "Male", 90.5);
Row row = sheet.getRow(1);
Cell cell = row.getCell(0);
cell.setCellValue(student.getName());
row.getCell(1).setCellValue(student.getAge());
row.getCell(2).setCellValue(student.getGender());
row.getCell(3).setCellValue(student.getScore());
// 保存文件
FileOutputStream outputStream = new FileOutputStream("output.xlsx");
workbook.write(outputStream);
outputStream.close();
workbook.close();
}
}
```
在上面的代码中,我们首先通过WorkbookFactory类加载Excel模板文件。然后,我们获取第一个Sheet,并使用实体类填充第二行的数据。最后,我们将修改后的Workbook保存为一个新的Excel文件。
阅读全文
相关推荐
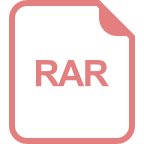
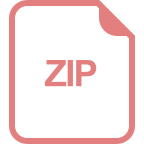
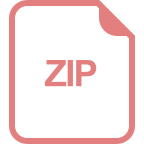
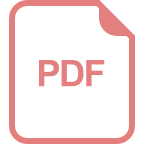
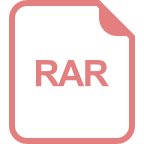
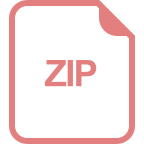
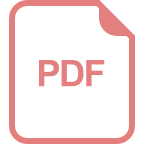
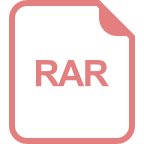
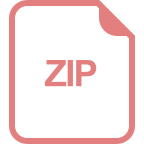
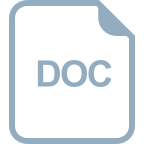
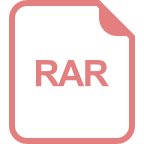
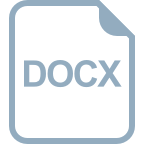
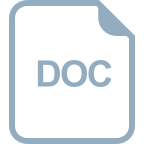
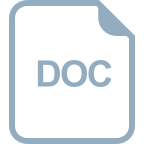
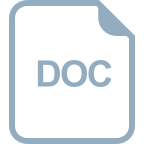
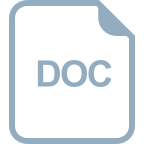