std::map插入数据
时间: 2023-07-20 15:30:31 浏览: 107
可以使用以下方式向 std::map 中插入数据:
```c++
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
// 使用 insert 函数插入一对 key-value 值
myMap.insert(std::pair<int, std::string>(1, "one"));
// 使用 [] 运算符插入一对 key-value 值
myMap[2] = "two";
// 使用 insert 函数插入多对 key-value 值
myMap.insert({
{3, "three"},
{4, "four"}
});
// 遍历 map 中的元素
for (const auto& pair : myMap) {
std::cout << pair.first << " : " << pair.second << std::endl;
}
return 0;
}
```
输出结果为:
```
1 : one
2 : two
3 : three
4 : four
```
相关问题
c++ std::undermap 插入相同数据
您好!关于您的问题,C++标准库中没有名为`std::undermap`的函数或类。可能是您想使用`std::unordered_map`,它是C++中的无序关联容器。在`std::unordered_map`中,插入相同的数据是不被允许的,每个键值对都应该是唯一的。
如果您尝试插入相同的键,则新值将替换旧值。例如:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap;
// 插入键值对
myMap.insert({1, "apple"});
myMap.insert({2, "banana"});
myMap.insert({3, "orange"});
// 尝试插入相同的键
myMap.insert({2, "grape"}); // 旧值 "banana" 将被替换为 "grape"
// 输出键值对
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
输出结果将是:
```
1: apple
2: grape
3: orange
```
如果您想要有重复的键值对,可以考虑使用`std::multimap`或自定义容器来实现。希望这能解答您的问题!如果您还有其他问题,请随时提问。
std::map<std::string, std::string> data 插入数据
你可以使用 `insert` 函数向 `std::map<std::string, std::string>` 中插入数据,示例代码如下:
```cpp
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, std::string> data;
data.insert(std::make_pair("key1", "value1"));
data.insert(std::make_pair("key2", "value2"));
data.insert(std::make_pair("key3", "value3"));
// 遍历 map 中的数据
for (const auto& item : data) {
std::cout << item.first << ": " << item.second << std::endl;
}
return 0;
}
```
以上代码中,我们首先通过 `std::map<std::string, std::string> data` 定义了一个名为 `data` 的 `std::map` 对象。然后我们使用 `insert` 函数向 `data` 中插入了三组数据,每组数据都是由键值对组成的。最后我们遍历了 `data` 中的所有数据并输出了它们。
阅读全文
相关推荐
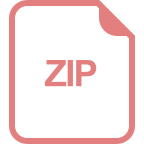
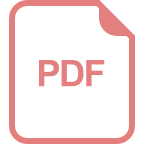
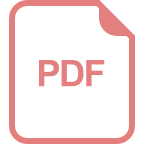


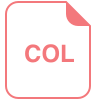










