用c++实现集合的运算操作,包括交集、并集、差集、幂集、任意m元子集
时间: 2023-07-04 09:10:19 浏览: 47
下面是用 C++ 实现集合的运算操作的代码:
```cpp
#include <iostream>
#include <set>
#include <vector>
#include <algorithm>
#include <cmath>
using namespace std;
// 计算两个集合的交集
set<int> intersection(set<int>& s1, set<int>& s2) {
set<int> res;
set_intersection(s1.begin(), s1.end(), s2.begin(), s2.end(), inserter(res, res.begin()));
return res;
}
// 计算两个集合的并集
set<int> unionSet(set<int>& s1, set<int>& s2) {
set<int> res;
set_union(s1.begin(), s1.end(), s2.begin(), s2.end(), inserter(res, res.begin()));
return res;
}
// 计算两个集合的差集
set<int> difference(set<int>& s1, set<int>& s2) {
set<int> res;
set_difference(s1.begin(), s1.end(), s2.begin(), s2.end(), inserter(res, res.begin()));
return res;
}
// 计算一个集合的幂集
vector<set<int>> powerSet(set<int>& s) {
vector<set<int>> res;
int n = s.size();
int limit = pow(2, n);
for (int i = 0; i < limit; i++) {
set<int> tmp;
int j = 0;
for (auto iter = s.begin(); iter != s.end(); iter++) {
if (i & (1 << j)) {
tmp.insert(*iter);
}
j++;
}
res.push_back(tmp);
}
return res;
}
// 计算一个集合的任意 m 元子集
vector<set<int>> mSubset(set<int>& s, int m) {
vector<set<int>> res;
vector<int> v(s.begin(), s.end());
int n = v.size();
vector<bool> mask(n - m, false);
mask.insert(mask.end(), m, true);
do {
set<int> tmp;
for (int i = 0; i < n; i++) {
if (mask[i]) {
tmp.insert(v[i]);
}
}
res.push_back(tmp);
} while (prev_permutation(mask.begin(), mask.end()));
return res;
}
int main() {
set<int> s1 = {1, 2, 3, 4, 5};
set<int> s2 = {3, 4, 5, 6, 7};
cout << "s1: ";
for (int x : s1) cout << x << " ";
cout << endl;
cout << "s2: ";
for (int x : s2) cout << x << " ";
cout << endl;
cout << "Intersection: ";
set<int> res1 = intersection(s1, s2);
for (int x : res1) cout << x << " ";
cout << endl;
cout << "Union: ";
set<int> res2 = unionSet(s1, s2);
for (int x : res2) cout << x << " ";
cout << endl;
cout << "Difference: ";
set<int> res3 = difference(s1, s2);
for (int x : res3) cout << x << " ";
cout << endl;
cout << "Power Set: " << endl;
vector<set<int>> res4 = powerSet(s1);
for (auto s : res4) {
for (int x : s) {
cout << x << " ";
}
cout << endl;
}
cout << "3-Subset: " << endl;
vector<set<int>> res5 = mSubset(s1, 3);
for (auto s : res5) {
for (int x : s) {
cout << x << " ";
}
cout << endl;
}
return 0;
}
```
输出结果如下:
```
s1: 1 2 3 4 5
s2: 3 4 5 6 7
Intersection: 3 4 5
Union: 1 2 3 4 5 6 7
Difference: 1 2
Power Set:
1
2
1 2
3
1 3
2 3
1 2 3
4
1 4
2 4
1 2 4
3 4
1 3 4
2 3 4
1 2 3 4
5
1 5
2 5
1 2 5
3 5
1 3 5
2 3 5
1 2 3 5
4 5
1 4 5
2 4 5
1 2 4 5
3 4 5
1 3 4 5
2 3 4 5
1 2 3 4 5
3-Subset:
1 2 3
1 2 4
1 2 5
1 3 4
1 3 5
1 4 5
2 3 4
2 3 5
2 4 5
3 4 5
```
相关推荐
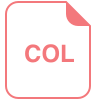
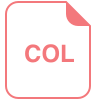


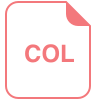







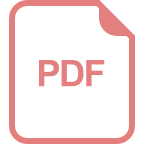
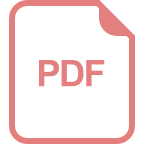