Spring Boot 中可以使用 JavaMail API 来读取 IMAP 邮件的附件并保存附件
时间: 2023-11-28 21:50:10 浏览: 218
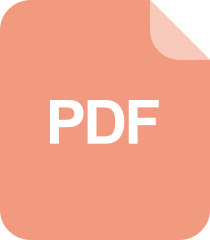
Spring Boot利用Java Mail实现邮件发送

是的,Spring Boot 中可以使用 JavaMail API 来读取 IMAP 邮件的附件并保存附件。JavaMail API 是 Java EE 平台中用于发送和接收电子邮件的标准 API,它提供了一组类和接口,使开发人员可以轻松地编写邮件客户端程序。
以下是一个示例代码,演示如何在 Spring Boot 中使用 JavaMail API 来读取 IMAP 邮件的附件并保存附件:
```java
import java.io.File;
import java.io.IOException;
import java.util.Properties;
import javax.mail.BodyPart;
import javax.mail.Flags;
import javax.mail.Folder;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.Session;
import javax.mail.Store;
import javax.mail.internet.MimeBodyPart;
import javax.mail.search.FlagTerm;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class MailReader {
@Value("${mail.username}")
private String username;
@Value("${mail.password}")
private String password;
@Value("${mail.imap.host}")
private String imapHost;
@Value("${mail.imap.port}")
private int imapPort;
public void readAndSaveAttachments() {
try {
Properties properties = new Properties();
properties.put("mail.imap.host", imapHost);
properties.put("mail.imap.port", imapPort);
properties.put("mail.imap.ssl.enable", "true");
Session session = Session.getDefaultInstance(properties);
Store store = session.getStore("imap");
store.connect(username, password);
Folder inbox = store.getFolder("INBOX");
inbox.open(Folder.READ_WRITE);
// Search for unread messages
FlagTerm flagTerm = new FlagTerm(new Flags(Flags.Flag.SEEN), false);
Message[] messages = inbox.search(flagTerm);
for (Message message : messages) {
Multipart multipart = (Multipart) message.getContent();
// Get all the attachments
for (int i = 0; i < multipart.getCount(); i++) {
BodyPart bodyPart = multipart.getBodyPart(i);
// If the bodypart is an attachment
if (bodyPart.getDisposition() != null && bodyPart.getDisposition().equalsIgnoreCase("attachment")) {
MimeBodyPart mimeBodyPart = (MimeBodyPart) bodyPart;
File file = new File(mimeBodyPart.getFileName());
// Save the attachment to a file
mimeBodyPart.saveFile(file);
}
}
// Mark the message as read
message.setFlag(Flags.Flag.SEEN, true);
}
inbox.close(false);
store.close();
} catch (MessagingException | IOException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们首先创建了一个 Properties 对象,用于配置连接到 IMAP 服务器的参数。然后,我们使用该 Properties 对象创建一个 Session 对象,并使用 Session 对象创建一个 Store 对象来连接到 IMAP 服务器。接下来,我们打开收件箱 Folder 对象,并使用 FlagTerm 对象搜索未读邮件。对于每个未读邮件,我们获取其内容并将其转换为 Multipart 对象。然后,我们遍历 Multipart 对象的所有 BodyPart 对象,并找到所有附件。对于每个附件,我们将其保存到一个文件中,并将消息标记为已读。
请注意,上面的代码中的用户名、密码、IMAP 主机和端口都是从应用程序的配置文件中读取的。在使用此代码时,请确保将这些值替换为您自己的值。
阅读全文
相关推荐
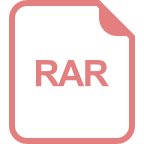

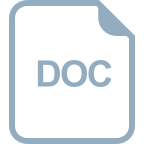
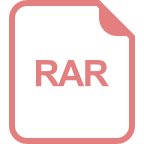
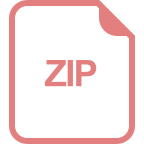


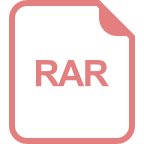
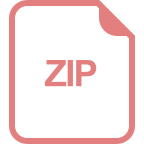
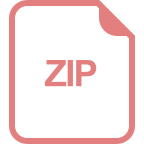
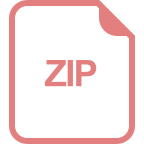
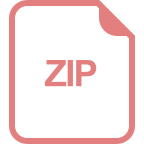