获取栈顶位置C语言代码
时间: 2023-12-11 10:56:26 浏览: 82
获取栈顶位置可以使用C语言中的指针操作。假设你使用的是系统自带的栈,可以通过以下代码获取栈顶位置:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
void *stack_top;
void *stack_bottom;
size_t stack_size;
// 获取栈顶指针
stack_top = &stack_top;
// 获取栈底指针
stack_bottom = &stack_bottom;
while (stack_bottom == &stack_bottom) {
stack_bottom++;
}
// 计算栈大小
stack_size = (size_t)stack_bottom - (size_t)stack_top;
printf("栈顶位置:%p\n", stack_top);
printf("栈底位置:%p\n", stack_bottom);
printf("栈大小:%zu\n", stack_size);
return 0;
}
```
该程序在不同的编译器和操作系统上可能存在一些差异,但通常是可行的。
相关问题
设计一个支持 push ,pop ,top 操作,并能在常数时间内检索到最小元素的栈。\n\npush(x) —— 将元素 x 推入栈中。\npop() —— 删除栈顶的元素。\ntop() —— 获取栈顶元素。C语言代码
设计这样的栈,我们可以采用一种叫做“带最小值的栈”(MinStack)的数据结构。它不仅包含常规的栈元素,还维护一个额外的指针来追踪当前栈中的最小元素。下面是 C 语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义 MinStack 结构体
typedef struct {
int* stack; // 栈数组
int top; // 栈顶元素索引
int min_index; // 最小值的索引
int size; // 当前大小
} MinStack;
// 初始化一个新的 MinStack
MinStack* createMinStack() {
MinStack* s = (MinStack*)malloc(sizeof(MinStack));
if (!s) return NULL;
s->stack = NULL;
s->top = -1;
s->min_index = -1;
s->size = 0;
return s;
}
// 压栈操作
void push(MinStack* s, int x) {
if (s->top == s->size - 1) {
s->stack = realloc(s->stack, (s->size *= 2) + sizeof(int));
if (!s->stack) {
free(s);
s = NULL;
return;
}
}
s->stack[++s->top] = x;
// 更新最小值
if (s->top == s->min_index || x <= s->stack[s->min_index]) {
s->min_index = s->top;
}
}
// 出栈操作
int pop(MinStack* s) {
if (s-1; // 空栈
int x = s->stack[s->top];
s->stack[s->top--] = 0; // 清空栈顶位置
// 如果删除的是最小值,更新最小值
if (x == s->stack[s->min_index]) {
if (s->top != s->min_index) {
s->min_index--;
} else {
while (s->top >= 0 && s->stack[s->top] > s->stack[s->min_index]) {
s->min_index++;
}
}
}
return x;
}
// 获取栈顶元素
int top(MinStack* s) {
return s->top == -1 ? -1 : s->stack[s->top];
}
// 查找并返回最小值
int getMin(MinStack* s) {
return s->stack[s->min_index];
}
// 释放内存
void destroyMinStack(MinStack* s) {
if (s && s->stack) free(s->stack);
free(s);
}
int main() {
MinStack* stack = createMinStack();
push(stack, -2);
push(stack, 0);
push(stack, -3);
printf("Top: %d, Min: %d\n", top(stack), getMin(stack)); // 输出:Top: 0, Min: -3
pop(stack);
printf("Top: %d, Min: %d\n", top(stack), getMin(stack)); // 输出:Top: -2, Min: -2
destroyMinStack(stack);
return 0;
}
```
这个实现中,`push`、`pop` 和 `top` 的操作时间复杂度都是 O(1),而 `getMin` 的时间复杂度也是 O(1),因为我们在压栈时就维护了最小值。
某一线性数据结构的数据集中的元素数据为不同城市的省会及其位置,数据元素类型定义为: typedef struct{ int city_id; double x; double y; double z; } ElemType; 通过创建链栈记录数据集,并实现销毁、入栈、出栈和获取栈顶元素的C语言代码实现。
好的,这是C语言实现某一线性数据结构的链栈操作的代码:
#include <stdio.h>
#include <stdlib.h>
typedef struct stack_node {
ElemType data;
struct stack_node *next;
} StackNode, *LinkStack;
// 初始化链栈
LinkStack InitStack() {
LinkStack top = (LinkStack)malloc(sizeof(StackNode));
top->next = NULL;
return top;
}
// 入栈操作
void Push(LinkStack top, ElemType data) {
StackNode *node = (StackNode*)malloc(sizeof(StackNode));
node->data = data;
node->next = top->next;
top->next = node;
}
// 出栈操作
ElemType Pop(LinkStack top) {
if (top->next == NULL) {
printf("The stack is empty!\n");
return;
}
StackNode *node = top->next;
top->next = node->next;
ElemType data = node->data;
free(node);
return data;
}
// 获取栈顶元素
ElemType GetTop(LinkStack top) {
if (top->next == NULL) {
printf("The stack is empty!\n");
return;
}
return top->next->data;
}
// 销毁链栈
void DestroyStack(LinkStack top) {
StackNode *p = top->next;
while (p != NULL) {
top->next = p->next;
free(p);
p = top->next;
}
free(top);
}
// 测试代码
int main() {
LinkStack top = InitStack();
ElemType e1 = {1, 1.0, 2.0, 3.0};
Push(top, e1);
ElemType e2 = {2, 4.0, 5.0, 6.0};
Push(top, e2);
ElemType e3 = {3, 7.0, 8.0, 9.0};
Push(top, e3);
printf("栈顶元素: city_id=%d, x=%lf, y=%lf, z=%lf\n", GetTop(top).city_id, GetTop(top).x, GetTop(top).y, GetTop(top).z);
ElemType e4 = Pop(top);
printf("出栈元素: city_id=%d, x=%lf, y=%lf, z=%lf\n", e4.city_id, e4.x, e4.y, e4.z);
DestroyStack(top);
return 0;
}
阅读全文
相关推荐
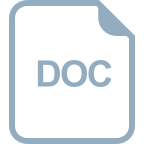



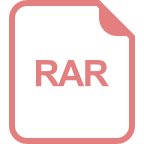
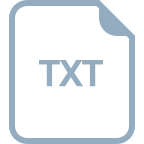
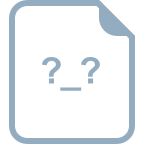






