led1与led2用t1定时10秒,5秒亮5秒灭,led1与led2状态相反。led3与led4用t3定时,产生20秒的方波,led3与led4状态相反。用cortex-m3编写代码。
时间: 2024-10-17 13:03:37 浏览: 15
在Cortex-M3微控制器上编写这样的代码通常需要利用中断、定时器和GPIO功能。这里是一个简单的示例,使用Keil MDK等IDE的C语言来实现:
```c
#include "stm32f1xx.h" // 引入STM32F1系列的头文件
// 定义LED GPIO口
GPIO_TypeDef *gpio LedPorts[] = {GPIOA, GPIOB}; // LED1, LED2
uint8_t LedPins[] = {GPIO_Pin_0, GPIO_Pin_1}; // LED1对应pin0, LED2对应pin1
// 定义定时器
TIM_HandleTypeDef Timers[2] = {TIM2, TIM3};
void initializeTimers(void) {
TIM_TimeBaseInitTypeDef TimerInitStruct;
TIM为空闲模式下配置TIM2:
TimerInitStruct.Init.Prescaler = ...; // 根据系统频率设置预分频值
TimerInitStruct.Init.CounterMode = TIM_COUNTERMODE_UP;
TimerInitStruct.Init.Period = 10*1000 - 1; // 10秒计数,减1避免溢出
HAL_TIM_Base_Init(&Timers[0], &TimerInitStruct);
HAL_TIM_Base_Start_IT(&Timers[0]); // 开启中断
// TIM3 方波配置
TimerInitStruct.Init.Prescaler = ...; // 另外一个合适的预分频值
TimerInitStruct.Init.Period = 1000; // 20秒周期
HAL_TIM_Base_Init(&Timers[1], &TimerInitStruct);
HAL_TIM_Base_Start_IT(&Timers[1]);
}
void toggleLED(uint8_t PortIndex, uint8_t PinIndex) {
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.Pin = LedPins[PinIndex];
GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; // 输出推挽模式
GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_VERY_HIGH;
HAL_GPIO_Init(LedPorts[PortIndex], &GPIO_InitStructure);
if (HAL_GPIO_ReadPin(LedPorts[PortIndex], LedPins[PinIndex]) == GPIO_PIN_RESET)
HAL_GPIO_WritePin(LedPorts[PortIndex], LedPins[PinIndex], GPIO_PIN_SET);
else
HAL_GPIO_WritePin(LedPorts[PortIndex], LedPins[PinIndex], GPIO_PIN_RESET);
}
void TIM_IRQHandler(uint16_t TimerNumber) {
static bool HalfPeriod = false;
if (TimerNumber == TIM2_IRQn) {
HAL_GPIO_TogglePin(LedPorts[0], LedPins[0]); // LED1动作
HAL_GPIO_TogglePin(LedPorts[1], LedPins[1]); // LED2动作
HalfPeriod = !HalfPeriod; // 切换状态
} else if (TimerNumber == TIM3_IRQn) {
if (!HalfPeriod) {
HAL_GPIO_TogglePin(LedPorts[2], LedPins[2]); // LED3动作
HAL_GPIO_TogglePin(LedPorts[3], LedPins[3]); // LED4动作
}
HalfPeriod = true;
}
HAL_TIM_IRQHandler(Timers[TimerNumber]);
}
int main(void) {
// 初始化硬件资源
HAL_Init();
SystemClock_Config();
initializeTimers();
while (1) {
// 进行其他任务...
}
}
阅读全文
相关推荐
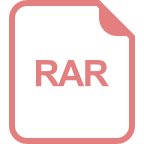
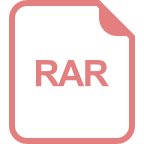
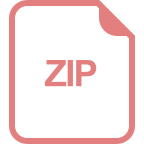















