c语言 银行排队系统 VIP和普通用户分别窗口
时间: 2024-04-28 07:20:19 浏览: 101
好的,C语言银行排队系统中,一般情况下会将VIP用户和普通用户分别安排到不同的窗口进行服务。这样可以提高VIP用户的优先服务体验,也能更好地管理人员和服务资源。
一种实现方式是在程序中设置两个队列,分别存放VIP用户和普通用户。当用户进入银行时,会根据其身份进行判断,如果是VIP用户则优先进入VIP队列,否则进入普通队列。然后根据队列的先进先出原则,依次安排用户到相应的窗口进行服务。同时,可以设置不同的服务时限和服务质量要求,进一步提高VIP用户的服务体验。
当然,这只是一种简单的实现方式,具体的实现方法还需要根据具体的需求和业务流程来确定。
相关问题
c语言 银行排队系统 VIP和普通用户分别窗口 代码
以下是一个简单的C语言银行排队系统的代码示例,其中包括了VIP和普通用户分别窗口的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义队列
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = 0;
q->rear = 0;
}
// 入队
void enQueue(Queue *q, int x) {
if ((q->rear + 1) % MAXSIZE == q->front) {
printf("Queue is full.\n");
exit(0);
}
q->data[q->rear] = x;
q->rear = (q->rear + 1) % MAXSIZE;
}
// 出队
int deQueue(Queue *q) {
if (q->front == q->rear) {
printf("Queue is empty.\n");
exit(0);
}
int x = q->data[q->front];
q->front = (q->front + 1) % MAXSIZE;
return x;
}
// 判断队列是否为空
int isEmpty(Queue q) {
return q.front == q.rear;
}
// 主函数
int main() {
Queue vipQueue, normalQueue;
initQueue(&vipQueue);
initQueue(&normalQueue);
int n, m;
printf("Please input the number of VIP users: ");
scanf("%d", &n);
printf("Please input the number of normal users: ");
scanf("%d", &m);
// VIP用户进入队列
for (int i = 0; i < n; i++) {
printf("Please input the arrival time and service time of VIP user %d: ", i+1);
int arrivalTime, serviceTime;
scanf("%d %d", &arrivalTime, &serviceTime);
if (isEmpty(vipQueue)) {
enQueue(&vipQueue, arrivalTime);
} else {
// 按照到达时间插入队列
int j = vipQueue.rear-1;
while (j >= vipQueue.front && vipQueue.data[j] > arrivalTime) {
vipQueue.data[j+1] = vipQueue.data[j];
j--;
}
vipQueue.data[j+1] = arrivalTime;
}
}
// 普通用户进入队列
for (int i = 0; i < m; i++) {
printf("Please input the arrival time and service time of normal user %d: ", i+1);
int arrivalTime, serviceTime;
scanf("%d %d", &arrivalTime, &serviceTime);
if (isEmpty(normalQueue)) {
enQueue(&normalQueue, arrivalTime);
} else {
// 按照到达时间插入队列
int j = normalQueue.rear-1;
while (j >= normalQueue.front && normalQueue.data[j] > arrivalTime) {
normalQueue.data[j+1] = normalQueue.data[j];
j--;
}
normalQueue.data[j+1] = arrivalTime;
}
}
// 开始服务
int currentTime = 0; // 当前时间
int vipIndex = 0; // VIP队列中等待服务的用户的下标
int normalIndex = 0; // 普通队列中等待服务的用户的下标
while (!isEmpty(vipQueue) || !isEmpty(normalQueue)) {
int vipArrivalTime = MAXSIZE, normalArrivalTime = MAXSIZE;
if (!isEmpty(vipQueue)) vipArrivalTime = vipQueue.data[vipQueue.front];
if (!isEmpty(normalQueue)) normalArrivalTime = normalQueue.data[normalQueue.front];
// 选择最早到达的用户
int arrivalTime = vipArrivalTime < normalArrivalTime ? vipArrivalTime : normalArrivalTime;
currentTime = arrivalTime;
// VIP用户优先服务
if (!isEmpty(vipQueue) && vipArrivalTime <= normalArrivalTime && vipQueue.data[vipIndex] <= currentTime) {
printf("VIP user %d is being serviced at VIP window.\n", vipIndex+1);
vipIndex++;
} else if (!isEmpty(normalQueue) && normalQueue.data[normalIndex] <= currentTime) {
printf("Normal user %d is being serviced at normal window.\n", normalIndex+1);
normalIndex++;
}
// 处理完服务时间最短的用户
if (!isEmpty(vipQueue) && vipArrivalTime <= normalArrivalTime && vipQueue.data[vipIndex-1] <= currentTime) {
deQueue(&vipQueue);
} else if (!isEmpty(normalQueue) && normalQueue.data[normalIndex-1] <= currentTime) {
deQueue(&normalQueue);
}
}
return 0;
}
```
以上代码仅供参考,具体实现方式和细节还需要根据实际需求进行调整和完善。
C语言银行排队系统问题
银行排队系统是一个常见的仿真问题,可以用C语言实现。以下是一个基本的单队列、多窗口的银行排队系统的实现,包括客户进入排队系统、客户离开、查询当前客户前面还有几人、查询截至目前总共办理多少客户等功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_QUEUE_SIZE 100 // 队列最大长度
#define MAX_WINDOW_NUM 10 // 窗口最大数量
typedef struct {
int id; // 客户编号
int type; // 客户类型,0表示普通客户,1表示VIP客户
int arrive_time; // 到达时间
int service_time; // 服务时间
int start_time; // 开始服务时间
int end_time; // 结束服务时间
} Customer;
typedef struct {
Customer data[MAX_QUEUE_SIZE]; // 队列数据
int front; // 队头指针
int rear; // 队尾指针
} Queue;
typedef struct {
int id; // 窗口编号
int type; // 窗口类型,0表示普通窗口,1表示VIP窗口
int busy; // 窗口是否忙碌,0表示空闲,1表示忙碌
int start_time; // 开始服务时间
int end_time; // 结束服务时间
} Window;
int customer_id = 0; // 客户编号计数器
int total_customer_num = 0; // 总共办理客户数量
int total_wait_time = 0; // 总共等待时间
int total_service_time = 0; // 总共服务时间
int window_num = 0; // 窗口数量
Window windows[MAX_WINDOW_NUM]; // 窗口数组
Queue queue; // 队列
// 初始化队列
void init_queue(Queue *q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int is_queue_empty(Queue *q) {
return q->front == q->rear;
}
// 判断队列是否已满
int is_queue_full(Queue *q) {
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
// 入队
void enqueue(Queue *q, Customer c) {
if (is_queue_full(q)) {
printf("Queue is full!\n");
return;
}
q->data[q->rear] = c;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
// 出队
Customer dequeue(Queue *q) {
if (is_queue_empty(q)) {
printf("Queue is empty!\n");
exit(1);
}
Customer c = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return c;
}
// 初始化窗口
void init_windows() {
for (int i = 0; i < window_num; i++) {
windows[i].id = i + 1;
windows[i].type = 0;
windows[i].busy = 0;
windows[i].start_time = 0;
windows[i].end_time = 0;
}
}
// 获取空闲窗口
int get_idle_window() {
for (int i = 0; i < window_num; i++) {
if (windows[i].busy == 0) {
return i;
}
}
return -1;
}
// 获取最早结束服务的窗口
int get_earliest_window() {
int earliest = 0;
for (int i = 1; i < window_num; i++) {
if (windows[i].end_time < windows[earliest].end_time) {
earliest = i;
}
}
return earliest;
}
// 获取VIP窗口
int get_vip_window() {
for (int i = 0; i < window_num; i++) {
if (windows[i].type == 1 && windows[i].busy == 0) {
return i;
}
}
return -1;
}
// 生成随机数
int random_int(int min, int max) {
return rand() % (max - min + 1) + min;
}
// 生成客户
Customer generate_customer(int arrive_time) {
Customer c;
c.id = ++customer_id;
c.type = random_int(0, 1);
c.arrive_time = arrive_time;
c.service_time = random_int(1, 10);
c.start_time = 0;
c.end_time = 0;
return c;
}
// 处理客户
void process_customer(Customer c) {
int idle_window = get_idle_window();
if (idle_window != -1) { // 有空闲窗口
windows[idle_window].busy = 1;
windows[idle_window].type = c.type;
windows[idle_window].start_time = c.arrive_time;
windows[idle_window].end_time = c.arrive_time + c.service_time;
c.start_time = windows[idle_window].start_time;
c.end_time = windows[idle_window].end_time;
total_service_time += c.service_time;
total_wait_time += c.start_time - c.arrive_time;
} else { // 没有空闲窗口,加入队列
enqueue(&queue, c);
}
}
// 处理窗口
void process_window(int current_time) {
for (int i = 0; i < window_num; i++) {
if (windows[i].busy && windows[i].end_time == current_time) { // 窗口服务结束
windows[i].busy = 0;
total_customer_num++;
if (!is_queue_empty(&queue)) { // 队列不为空,取出队首客户进行服务
Customer c = dequeue(&queue);
windows[i].busy = 1;
windows[i].type = c.type;
windows[i].start_time = current_time;
windows[i].end_time = current_time + c.service_time;
c.start_time = windows[i].start_time;
c.end_time = windows[i].end_time;
total_service_time += c.service_time;
total_wait_time += c.start_time - c.arrive_time;
}
}
}
}
// 打印客户信息
void print_customer(Customer c) {
printf("Customer %d: type=%d, arrive_time=%d, service_time=%d, start_time=%d, end_time=%d\n",
c.id, c.type, c.arrive_time, c.service_time, c.start_time, c.end_time);
}
// 打印窗口信息
void print_window(Window w) {
printf("Window %d: type=%d, busy=%d, start_time=%d, end_time=%d\n",
w.id, w.type, w.busy, w.start_time, w.end_time);
}
// 打印客户队列信息
void print_queue(Queue *q) {
printf("Queue: ");
for (int i = q->front; i != q->rear; i = (i + 1) % MAX_QUEUE_SIZE) {
printf("%d ", q->data[i].id);
}
printf("\n");
}
// 打印窗口信息
void print_windows() {
for (int i = 0; i < window_num; i++) {
print_window(windows[i]);
}
}
// 打印排队系统信息
void print_system(int current_time) {
printf("Current time: %d\n", current_time);
printf("Total customer num: %d\n", total_customer_num);
printf("Total wait time: %d\n", total_wait_time);
printf("Total service time: %d\n", total_service_time);
printf("Average wait time: %.2f\n", (float) total_wait_time / total_customer_num);
printf("Window status:\n");
print_windows();
printf("Queue status:\n");
print_queue(&queue);
}
int main() {
srand(time(NULL));
printf("Please input the number of windows: ");
scanf("%d", &window_num);
init_windows();
init_queue(&queue);
int current_time = 0;
while (current_time < 480) { // 一天的运行时间为480分钟
Customer c = generate_customer(current_time);
printf("Customer %d arrives at %d, type=%d, service_time=%d\n",
c.id, c.arrive_time, c.type, c.service_time);
process_customer(c);
print_system(current_time);
process_window(current_time);
current_time++;
}
printf("Simulation ends.\n");
return 0;
}
```
阅读全文
相关推荐
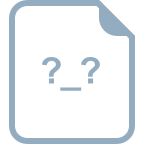
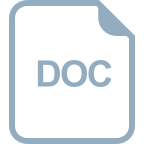
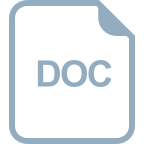
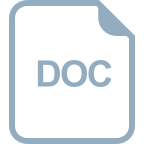
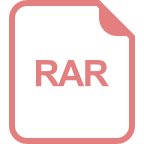
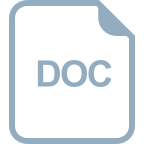
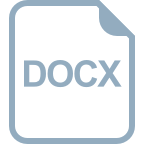
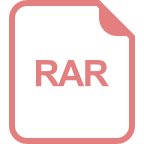
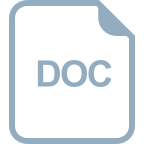
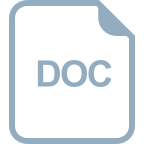



