如何用get_s代替gets
时间: 2024-05-10 12:18:27 浏览: 87
首先,建议使用更安全的 `fgets` 代替 `gets`。
而如果想要使用 `get_s` (即 `gets_s`),可以按照以下步骤:
1. 在代码中添加头文件 `<stdio.h>` 和 `<stdlib.h>`。
2. 使用 `fgets` 函数读取用户输入的数据。例如:
```c
char str[100];
fgets(str, sizeof(str), stdin);
```
3. 如果需要去掉字符串末尾的换行符,可以使用 `strcspn` 函数。例如:
```c
str[strcspn(str, "\n")] = '\0';
```
这个函数会返回第一个匹配 `\n` 的字符在字符串中的位置,然后我们用 `\0` 将其替换掉,这样就去掉了换行符。
4. 如果需要将输入转换为数字等其他类型,可以使用 `sscanf` 函数。例如:
```c
int num;
sscanf(str, "%d", &num);
```
这个函数会将 `str` 中的内容按照指定的格式读取到 `num` 中。
需要注意的是,`fgets` 和 `sscanf` 函数都需要指定读取的长度或格式,以防止缓冲区溢出等问题。
相关问题
优化@app.route('/api/verbal_trick/gets', methods=['get']) def verbal_trick_gets(): # 状态 type = request.args.get("type") type= map_type(type) if not type and type != 0: message = {"status": "failure", "content": "没有监测到传输的类型"} print(f"异常返回{message}") return jsonify(message) if int(type) in (0, 1, 2, 3, 4, 5, 6): mydb = dbUtil() # 执行 SQL 查询 mycursor = mydb.cursor() query = "select * from ai_livechat_table where type =%d order by priority " % int(type) mycursor.execute(query) # 获取查询结果 mylist = [] myresult = mycursor.fetchall() for row in myresult: mydict = {"id": row[0], "question": row[1], "createtime": row[2], "type": row[4], "priority": row[5]} mylist.append(mydict) message = {"status": "succeed", "content": mylist} return jsonify(message) else: message = {"status": "failure", "content": "没有此种类型"} print(f"异常返回{message}") return jsonify(message)
可以考虑以下优化:
1. 使用 try-except 语句来捕获异常,而不是直接使用 if 语句来判断是否有传输类型。这样可以更加准确地捕获异常,同时也可以避免代码重复。
2. 使用参数化查询来代替字符串拼接查询语句。这样可以更加安全地执行 SQL 查询,避免 SQL 注入攻击。
3. 使用 with 语句来代替手动关闭数据库连接。这样可以更加简洁地管理数据库连接,同时也可以避免忘记关闭连接导致的资源泄漏。
下面是优化后的代码:
```
@app.route('/api/verbal_trick/gets', methods=['get'])
def verbal_trick_gets():
try:
# 获取传输类型
type = int(request.args.get("type", -1))
type = map_type(type)
if type not in (0, 1, 2, 3, 4, 5, 6):
raise ValueError("没有此种类型")
# 执行 SQL 查询
with dbUtil() as mydb:
mycursor = mydb.cursor()
query = "SELECT * FROM ai_livechat_table WHERE type = %s ORDER BY priority"
mycursor.execute(query, (type,))
myresult = mycursor.fetchall()
# 构造返回结果
mylist = [{"id": row[0], "question": row[1], "createtime": row[2], "type": row[4], "priority": row[5]} for row in myresult]
message = {"status": "succeed", "content": mylist}
return jsonify(message)
except Exception as e:
message = {"status": "failure", "content": str(e)}
print(f"异常返回{message}")
return jsonify(message)
```
c语言get函数怎么用??
在C语言中,`get`函数通常是指 `gets()` 函数,但请注意,这个函数已经被认为是不安全的,因为它不会检查输入长度可能导致缓冲区溢出。因此,在现代标准库中,推荐使用更安全的 `fgets()` 或 `scanf()` 代替。
如果你想获取用户从标准输入流(如键盘)输入的一行文本,可以使用 `fgets()` 函数。它的基本用法如下:
```c
#include <stdio.h>
#define MAX_LINE_LENGTH 100 // 设置最大输入长度
int main() {
char input[MAX_LINE_LENGTH]; // 存储用户输入的字符数组
printf("Please enter something: ");
fgets(input, MAX_LINE_LENGTH, stdin); // 第二个参数指定接收的最大字符数,包括换行符
input[strcspn(input, "\n")] = '\0'; // 移除换行符,防止后续处理出现问题
printf("You entered: %s\n", input);
return 0;
}
```
这里,`fgets()` 接收三个参数:目标数组、最大存储长度(不包含结束符 `\0`)以及输入流(通常为 `stdin` 表示标准输入)。
`scanf()` 可以配合格式说明符 `%s` 直接读取字符串,但它也不能处理换行符,所以通常需要额外处理。
阅读全文
相关推荐
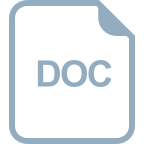
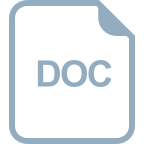
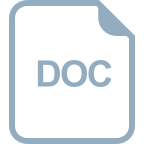
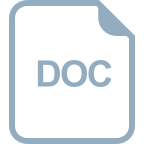

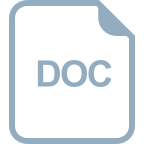
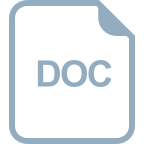
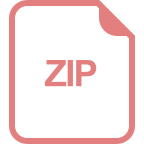
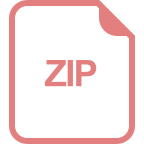
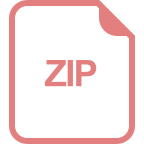
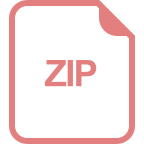
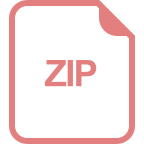