用c语言的栈的顺序存储结构及其基本操作编写程序,对于输入的任意一个非负十进制整数,输出与其等值的八进制数。
时间: 2023-06-18 18:07:44 浏览: 71
以下是用C语言实现栈的顺序存储结构及其基本操作的程序,以及将输入的非负十进制整数转换为八进制数的程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define STACK_INIT_SIZE 100
#define STACKINCREMENT 10
typedef struct {
int *base;
int *top;
int stacksize;
} SqStack;
void InitStack(SqStack *S) {
S->base = (int *) malloc(STACK_INIT_SIZE * sizeof(int));
if (!S->base) {
exit(0);
}
S->top = S->base;
S->stacksize = STACK_INIT_SIZE;
}
void Push(SqStack *S, int e) {
if (S->top - S->base >= S->stacksize) {
S->base = (int *) realloc(S->base, (S->stacksize + STACKINCREMENT) * sizeof(int));
if (!S->base) {
exit(0);
}
S->top = S->base + S->stacksize;
S->stacksize += STACKINCREMENT;
}
*(S->top) = e;
S->top++;
}
int Pop(SqStack *S, int *e) {
if (S->top == S->base) {
return 0;
}
*e = *(--S->top);
return 1;
}
int StackEmpty(SqStack *S) {
if (S->top == S->base) {
return 1;
} else {
return 0;
}
}
int main() {
SqStack S;
int n, e;
printf("请输入一个非负十进制整数:");
scanf("%d", &n);
InitStack(&S);
while (n > 0) {
Push(&S, n % 8);
n /= 8;
}
printf("八进制数为:");
while (!StackEmpty(&S)) {
Pop(&S, &e);
printf("%d", e);
}
printf("\n");
return 0;
}
```
输入一个非负十进制整数,程序使用栈的顺序存储结构将其转换为八进制数并输出。
相关推荐
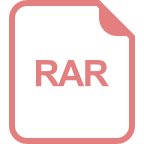














