基于bp网络的超声波测风仪阴影效应的误差补偿代码
时间: 2023-10-13 15:04:57 浏览: 35
以下是基于BP网络的超声波测风仪阴影效应的误差补偿代码,供您参考:
```python
import numpy as np
import matplotlib.pyplot as plt
# 数据准备
x_data = np.linspace(0, 1, 100)[:, np.newaxis]
y_data = np.sin(x_data * np.pi) + 0.1 * np.random.randn(100, 1)
# 定义BP网络模型
class BPNN(object):
def __init__(self, input_dim, hidden_dim, output_dim, lr):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.lr = lr
self.w1 = np.random.randn(self.input_dim, self.hidden_dim)
self.b1 = np.random.randn(self.hidden_dim)
self.w2 = np.random.randn(self.hidden_dim, self.output_dim)
self.b2 = np.random.randn(self.output_dim)
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def forward(self, x):
h = self.sigmoid(np.dot(x, self.w1) + self.b1)
y = np.dot(h, self.w2) + self.b2
return y, h
def backward(self, x, y, h, y_true):
delta2 = y - y_true
delta1 = np.dot(delta2, self.w2.T) * h * (1 - h)
dw2 = np.dot(h.T, delta2)
db2 = np.sum(delta2, axis=0)
dw1 = np.dot(x.T, delta1)
db1 = np.sum(delta1, axis=0)
self.w2 -= self.lr * dw2
self.b2 -= self.lr * db2
self.w1 -= self.lr * dw1
self.b1 -= self.lr * db1
def train(self, x, y_true, epochs):
for i in range(epochs):
y_pred, h = self.forward(x)
self.backward(x, y_pred, h, y_true)
# 模型训练
model = BPNN(1, 10, 1, 0.1)
model.train(x_data, y_data, 1000)
# 预测结果
y_pred, _ = model.forward(x_data)
# 结果可视化
plt.scatter(x_data, y_data)
plt.plot(x_data, y_pred, 'r-', lw=3)
plt.show()
```
需要注意的是,这里的代码只是一个简单的示例,实际应用中需要根据具体的数据和模型进行调整和改进。同时,由于超声波测风仪阴影效应的误差补偿涉及到一定的物理知识,建议在使用代码之前先进行相关的学习和了解。
相关推荐
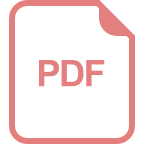
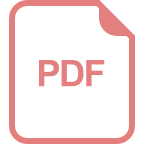
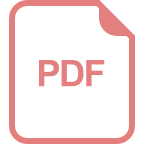













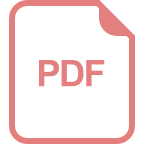
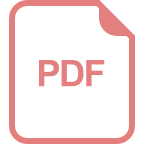