java读取MP3并播放MP3
时间: 2023-10-18 07:18:26 浏览: 105
要播放MP3文件,可以使用Java的Java Sound API。以下是一个简单的示例代码,演示如何读取并播放MP3文件:
```java
import javax.sound.sampled.*;
import java.io.*;
public class MP3Player {
public static void main(String[] args) {
try {
// 打开MP3文件
File file = new File("music.mp3");
AudioInputStream in = AudioSystem.getAudioInputStream(file);
// 获取音频格式
AudioFormat format = in.getFormat();
// 转换音频格式为PCM编码
AudioFormat pcm = new AudioFormat(
AudioFormat.Encoding.PCM_SIGNED,
format.getSampleRate(),
16,
format.getChannels(),
format.getChannels() * 2,
format.getSampleRate(),
false);
// 获取转换后的音频流
AudioInputStream din = AudioSystem.getAudioInputStream(pcm, in);
// 获取音频输出设备
DataLine.Info info = new DataLine.Info(SourceDataLine.class, pcm);
SourceDataLine line = (SourceDataLine) AudioSystem.getLine(info);
line.open(pcm);
line.start();
// 播放音频
byte[] buffer = new byte[4096];
int bytesRead = 0;
while ((bytesRead = din.read(buffer, 0, buffer.length)) != -1) {
line.write(buffer, 0, bytesRead);
}
// 关闭流
din.close();
in.close();
line.drain();
line.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
注意,这个代码需要引入Java Sound API的库,可以在Java SDK的lib目录下找到。
相关推荐
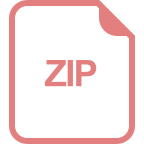














