定义单链表的结点类型 struct node { int val; node *next; }。 定义函数 node *insert(node *h,node *p) 用于将p指向的结点升序插入在升序链表h中,返回新链表头指针。 定义函数 node *create( ) 用于创建链表,函数内不断输入整数(^Z结束)构成链表结点(动态结点),返回链表头指针。 定义函数 void print(node *h) 用于输出链表h的值,每个值后面跟两个空格,最后换行。 定义函数 node *delNode(node *h,int x) 用于在链表h中删除第一个值为x的结点(若存在),返回链表头指针 定义函数 void delAll(node *h) 用于删除整个链表,释放链表结点占用的动态空间。 主函数调用 create 创建一个升序单链表head,调用 print 输出该链表,然后调用 delNode 删除链表head中第一个值为100的结点,再调用 insert 在链表中升序插入结点,该结点的值为200,最后调用 print 在下一行输出整个链表。输出的两行数据中,每个数据后面跟两个空格。
时间: 2023-06-16 20:02:18 浏览: 49
```c++
#include <iostream>
using namespace std;
struct node {
int val;
node *next;
};
node *create() {
node *head = NULL, *tail = NULL;
int x;
while (cin >> x) {
node *p = new node;
p->val = x;
p->next = NULL;
if (!head) head = tail = p;
else {
tail->next = p;
tail = p;
}
}
return head;
}
void print(node *h) {
while (h) {
cout << h->val << " ";
h = h->next;
}
cout << endl;
}
node *delNode(node *h, int x) {
if (!h) return NULL;
if (h->val == x) {
node *p = h->next;
delete h;
return p;
}
node *pre = h, *cur = h->next;
while (cur) {
if (cur->val == x) {
pre->next = cur->next;
delete cur;
break;
}
pre = cur;
cur = cur->next;
}
return h;
}
node *insert(node *h, node *p) {
if (!h) return p;
if (p->val < h->val) {
p->next = h;
return p;
}
node *pre = h, *cur = h->next;
while (cur) {
if (p->val < cur->val) {
p->next = cur;
pre->next = p;
break;
}
pre = cur;
cur = cur->next;
}
if (!cur) pre->next = p;
return h;
}
void delAll(node *h) {
while (h) {
node *p = h;
h = h->next;
delete p;
}
}
int main() {
node *head = create();
print(head);
head = delNode(head, 100);
node *p = new node;
p->val = 200;
p->next = NULL;
head = insert(head, p);
print(head);
delAll(head);
return 0;
}
```
相关推荐
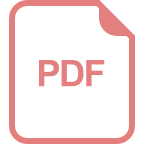
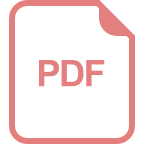
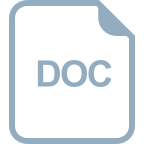














