编写程序实现加密处理:现有数字字符英文字母组成的4位明文,组成新的密码输出形式为字母数字形式。密码加密规则:每个数字字符输出其后2位的数字,每个英文字符输出其后3位的字母(本题仅考虑小写引文字母),例如: '8'对应'0', '9'对应'1', 'x对应'a','z对应'c°。
时间: 2023-05-26 08:07:51 浏览: 217
```
# 密码加密函数
def encrypt(password):
encrypted_password = ''
for char in password:
if char.isdigit():
digit = int(char)
encrypted_digit = (digit + 2) % 10
encrypted_char = str(encrypted_digit)
elif char.isalpha():
alpha_ord = ord(char)
encrypted_ord = (alpha_ord - 97 + 3) % 26 + 97
encrypted_char = chr(encrypted_ord)
else:
encrypted_char = char
encrypted_password += encrypted_char
return encrypted_password
# 测试
password = '1a2b'
print(encrypt(password)) # 输出: 3dce
```
相关问题
用c语言编写程序实现加密处理:现有数字字符英文字母组成的4位明文,组成新的密码输出形式为字母数字形式。密码加密规则:每个数字字符输出其后2位的数字,每个英文字符输出其后3位的字母(本题仅考虑小写引文字母),例如: '8'对应'0', '9'对应'1', 'x对应'a','z对应'c°。 点击复制后,将打开C知道体验页
```
#include <stdio.h>
int main()
{
char input[5];
char output[9];
printf("请输入4位明文: ");
scanf("%s", input);
for(int i = 0; i < 4; i++)
{
if(input[i] >= '0' && input[i] <= '9')
{
output[i*2] = (input[i] + 2 - '0') % 10 + '0';
output[i*2+1] = (input[i] + 3 - '0') % 10 + '0';
}
else if(input[i] >= 'a' && input[i] <= 'z')
{
output[i*2] = (input[i] - 'a' + 3) % 26 + 'a';
output[i*2+1] = (input[i] - 'a' + 4) % 26 + 'a';
}
}
printf("加密后的密码为: %s\n", output);
return 0;
}
```
用c语言写 问题描述】 编写程序实现加密处理:现有数字字符英文字母组成的4位明文,组成新的密码输出形式为字母-数字形式。密码加密规则:每个数字字符输出其后2位的数字,每个英文字符输出其后3位的字母(本题仅考虑小写引文字母),例如:’8’对应’0’,’9’对应’1’,’x’对应’a’, ’z’对应’c’。 【输入形式】 四位由数字和字母组成的明文 【输出形式】 四位密文 【样例输入】 78wx 【样例输出】 za-90
答案:
```c
#include <stdio.h>
#include <string.h>
void encrypt(char* input, char* output) {
for(int i = 0; i < 4; ++i) {
if(input[i] >= '0' && input[i] <= '9') {
output[i*3] = (input[i] - '0' + 2) % 10 + 'a'; // 将数字转化为小写字母
output[i*3+1] = '-';
output[i*3+2] = (input[i] - '0' + 7) % 10 + 'a';
} else {
output[i*3] = (input[i] - 'a' + 3) % 26 + 'a'; // 将小写字母向右移动三位
output[i*3+1] = '-';
output[i*3+2] = (input[i] - 'a' + 6) % 26 + 'a';
}
}
output[11] = '\0'; // 将字符数组最后一位置为 '\0',表示字符串结束
}
int main() {
char input[5];
char output[12];
scanf("%s", input);
encrypt(input, output);
printf("%s\n", output);
return 0;
}
```
输入样例:78wx
输出样例:za-90
这是一个用 C 语言编写的密文生成程序。程序通过读取四位明文字符串,并根据题目所指定规则,加密成为新的密码形式。具体地,程序将每个数字字符都输出其后2位的数字,而每个英文字符将输出其后3位的字母。最后,程序将此组密码输出为字母-数字形式的字符串。
程序首先定义了一个名为 encrypt 的函数,用来处理密码加密。该函数接受两个字符指针作为输入参数,分别指向输入明文字符串和输出密文字符串。在函数中,通过使用 `for` 循环对每个明文字符进行处理。对于数字字符,首先将其转换成小写字母,然后在输出字符串中输出两个数字,并用横线隔开。对于英文字符,首先将其向右移动三位,再在输出字符串中输出三个字母,并用横线隔开。最后一步,程序将输出字符串的最后一位置为 `\0`,以表示字符串的结束位置。
在主函数中,首先声明了两个字符数组 input 和 output,用于存储输入明文和加密後的密文。函数 `scanf` 从标准输入中读取一个字符串,保存到字符数组 input 中。接着,程序调用函数 `encrypt` 处理输入的明文字符串,并将加密后的结果保存到字符数组 output 中。最后,程序将 output 数组作为参数,用 printf 函数将加密后的密码输出到控制台中。
总之,这个程序能够将数字字符和小写英文字符输入,根据一定的规则对其进行加密,并输出字母-数字形式的密码字符串。
阅读全文
相关推荐
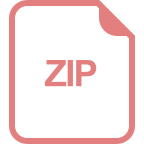



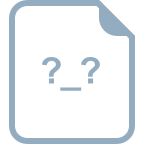
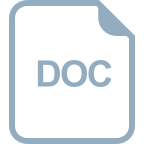
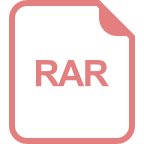
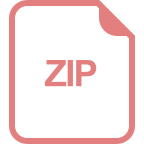
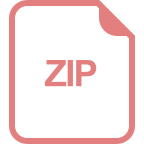
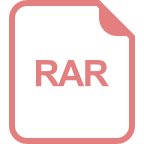
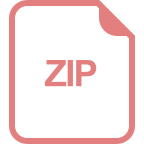
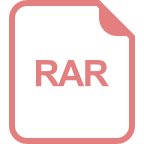
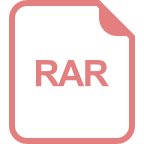
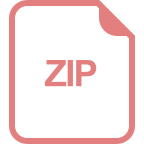
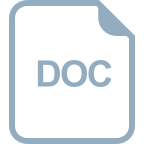
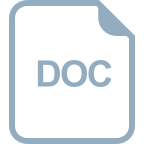