AlertDialog.setView()怎样添加一个textview一个edittext
时间: 2023-06-05 17:08:14 浏览: 42
可以使用以下代码实现:
```
AlertDialog.Builder builder = new AlertDialog.Builder(context);
View view = LayoutInflater.from(context).inflate(R.layout.layout_dialog, null);
TextView textView = view.findViewById(R.id.textView);
EditText editText = view.findViewById(R.id.editText);
builder.setView(view);
```
其中,R.layout.layout_dialog 表示包含 TextView 和 EditText 的布局文件,需要自己创建,可以在其中设置对应的 id,如上面的 R.id.textView 和 R.id.editText。同时,需要在使用前将 context 替换成实际的上下文对象。
相关问题
public void text2(){ View layout=View.inflate(this,R.layout.dialog_username,null); AlertDialog.Builder b=new AlertDialog.Builder(this); b.setTitle("修改名称"); b.setView(layout); b.setPositiveButton("确定", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int which) { EditText update=layout.findViewById(R.id.view2); SharedPreferences sp1 = getSharedPreferences("mydata", Context.MODE_PRIVATE); SharedPreferences.Editor editor1 = sp1.edit(); editor1.putString("USER", update.getText().toString()); editor1.commit(); Toast.makeText(ziliaoye02.this,"保存成功!", Toast.LENGTH_LONG).show(); finish(); String USER = sp1.getString("USER", ""); view2.setText(USER); //String newName=update.getText().toString().trim(); //view2.setText(newName); } }); b.setNegativeButton("取消",null); b.show(); }
这段代码是一个函数,名为 `text2()`,它创建了一个对话框来修改用户名,并将新的用户名保存在 `SharedPreferences` 中。下面是代码的解释:
1. `View layout=View.inflate(this,R.layout.dialog_username,null);`
创建了一个视图 `layout`,它使用 `dialog_username.xml` 中定义的布局。这个布局包含一个文本框 `EditText` 和一个文本视图 `TextView`。
2. `AlertDialog.Builder b=new AlertDialog.Builder(this);`
创建了一个对话框构建器 `b`,它将用于创建对话框。
3. `b.setTitle("修改名称");`
设置对话框的标题为 "修改名称"。
4. `b.setView(layout);`
将视图 `layout` 设置为对话框的内容。
5. `b.setPositiveButton("确定", new DialogInterface.OnClickListener() { ... }`
设置对话框的 "确定" 按钮的点击事件监听器。当用户点击 "确定" 时,将执行监听器中的代码。
6. `EditText update=layout.findViewById(R.id.view2);`
获取视图 `layout` 中的文本框 `EditText`,并将其赋值给变量 `update`。
7. `SharedPreferences sp1 = getSharedPreferences("mydata", Context.MODE_PRIVATE);`
获取名为 "mydata" 的 `SharedPreferences` 实例,使用私有模式(只能被当前应用程序访问)。
8. `SharedPreferences.Editor editor1 = sp1.edit();`
获取 `SharedPreferences` 的编辑器 `editor1`,用于修改 `SharedPreferences` 中的数据。
9. `editor1.putString("USER", update.getText().toString());`
将变量 `update` 中的文本框内容保存到 `SharedPreferences` 中,键为 "USER"。
10. `editor1.commit();`
提交 `SharedPreferences` 的修改。
11. `Toast.makeText(ziliaoye02.this,"保存成功!", Toast.LENGTH_LONG).show();`
显示 "保存成功!" 的消息提示。
12. `finish();`
结束当前活动(Activity),返回上一个活动。
13. `String USER = sp1.getString("USER", "");`
从 `SharedPreferences` 中获取键为 "USER" 的字符串值,并将其赋值给变量 `USER`。
14. `view2.setText(USER);`
将变量 `USER` 中的字符串值显示在文本视图 `TextView` 中。
以上就是这段代码的主要逻辑。它实现了一个简单的修改用户名的对话框,并将新的用户名保存在 `SharedPreferences` 中。
android在onCreateDialog中使用AlertDialog 加载设置自定义一个弹窗布局完整xml与java代码
以下是一个使用AlertDialog加载自定义布局的示例代码:
```java
public class MainActivity extends AppCompatActivity {
private Button showDialogBtn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//获取弹窗按钮
showDialogBtn = findViewById(R.id.show_dialog_btn);
//设置点击事件
showDialogBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//加载自定义布局
LayoutInflater inflater = LayoutInflater.from(MainActivity.this);
View dialogView = inflater.inflate(R.layout.custom_dialog_layout, null);
//创建AlertDialog
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle("自定义弹窗");
builder.setView(dialogView);
//设置确认按钮点击事件
builder.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
//处理确认按钮点击事件
}
});
//设置取消按钮点击事件
builder.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
//处理取消按钮点击事件
}
});
//显示弹窗
AlertDialog dialog = builder.create();
dialog.show();
}
});
}
}
```
其中,`R.layout.custom_dialog_layout` 为自定义的弹窗布局。你可以根据自己的需求来设计布局。在此仅提供一个示例布局,如下所示:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="请输入用户名和密码" />
<EditText
android:id="@+id/username_et"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="用户名" />
<EditText
android:id="@+id/password_et"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="密码"
android:inputType="textPassword" />
</LinearLayout>
```
相关推荐
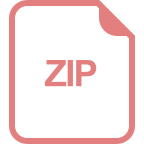
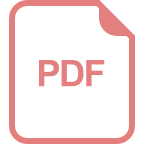
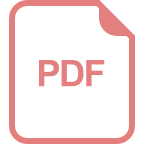












