可以用C语言代码实现建立链栈,并在链栈上实现入栈和出栈操作么
时间: 2024-02-01 11:13:59 浏览: 24
可以的,以下是用C语言实现链栈的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链栈结构体
typedef struct node {
int data;
struct node *next;
} Node, *Stack;
// 初始化链栈
void initStack(Stack *s) {
*s = NULL;
}
// 判断链栈是否为空
int isEmpty(Stack s) {
return s == NULL;
}
// 入栈操作
void push(Stack *s, int x) {
Node *p = (Node *)malloc(sizeof(Node));
p->data = x;
p->next = *s;
*s = p;
}
// 出栈操作
int pop(Stack *s) {
if (*s == NULL) {
printf("栈空,无法出栈\n");
return -1;
}
int x = (*s)->data;
Node *p = *s;
*s = (*s)->next;
free(p);
return x;
}
int main() {
Stack s;
initStack(&s);
push(&s, 1);
push(&s, 2);
push(&s, 3);
while (!isEmpty(s)) {
printf("%d ", pop(&s));
}
printf("\n");
return 0;
}
```
在上述代码中,我们定义了一个链栈的结构体 `Node`,并在入栈操作 `push` 和出栈操作 `pop` 中,利用头插法实现了链栈的入栈和出栈。在 `push` 操作中,我们首先申请一个新节点 `p`,将数据 `x` 存入其中,然后将 `p` 插入到栈顶 `s` 中;而在 `pop` 操作中,我们则是从栈顶 `s` 中取出一个节点 `p`,将其数据 `x` 返回,并释放其空间。
最后,在 `main` 函数中,我们演示了链栈的基本操作,包括初始化 `initStack`、入栈 `push`、出栈 `pop` 等。
相关推荐
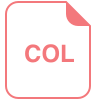
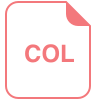












