用c语言建立链栈,并在链栈上实现入栈和出栈操作
时间: 2024-02-03 17:14:56 浏览: 90
好的,下面是C语言实现链栈的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链栈结构体
typedef struct node {
int data;
struct node *next;
} Node, *LinkStack;
// 初始化链栈
void InitStack(LinkStack *S) {
*S = NULL;
}
// 判断链栈是否为空
int IsEmpty(LinkStack S) {
return (S == NULL);
}
// 入栈操作
void Push(LinkStack *S, int x) {
Node *p = (Node*)malloc(sizeof(Node));
p->data = x;
p->next = *S;
*S = p;
}
// 出栈操作
int Pop(LinkStack *S) {
if (IsEmpty(*S)) {
printf("Stack is empty.");
return -1;
} else {
Node *p = *S;
int x = p->data;
*S = p->next;
free(p);
return x;
}
}
int main() {
LinkStack S; // 定义一个链栈
InitStack(&S); // 初始化链栈
// 进行入栈操作
Push(&S, 1);
Push(&S, 2);
Push(&S, 3);
// 进行出栈操作
printf("%d\n", Pop(&S));
printf("%d\n", Pop(&S));
printf("%d\n", Pop(&S));
return 0;
}
```
以上代码中,我们定义了一个链栈结构体Node,并定义了链栈的初始化、判断是否为空、入栈和出栈操作。在主函数中,我们初始化链栈并进行入栈和出栈操作,最后输出结果。
阅读全文
相关推荐





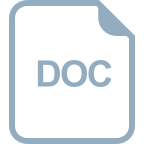
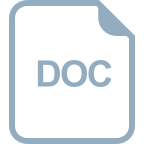







